- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter

JavaScript Assignment Operators
A ssignment operators.
Assignment operators are used to assign values to variables in JavaScript.
Assignment Operators List
There are so many assignment operators as shown in the table with the description.
OPERATOR NAME | SHORTHAND OPERATOR | MEANING |
---|---|---|
a+=b | a=a+b | |
a-=b | a=a-b | |
a*=b | a=a*b | |
a/=b | a=a/b | |
a%=b | a=a%b | |
a**=b | a=a**b | |
a<<=b | a=a<<b | |
a>>=b | a=a>>b | |
a&=b | a=a&b | |
a|=b | a=a | b | |
a^=b | a=a^b | |
| a&&=b | x && (x = y) |
| ||= | x || (x = y) |
| ??= | x ?? (x = y) |
Below we have described each operator with an example code:
Addition assignment operator(+=).
The Addition assignment operator adds the value to the right operand to a variable and assigns the result to the variable. Addition or concatenation is possible. In case of concatenation then we use the string as an operand.
Subtraction Assignment Operator(-=)
The Substraction Assignment Operator subtracts the value of the right operand from a variable and assigns the result to the variable.
Multiplication Assignment Operator(*=)
The Multiplication Assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable.
Division Assignment Operator(/=)
The Division Assignment operator divides a variable by the value of the right operand and assigns the result to the variable.
Remainder Assignment Operator(%=)
The Remainder Assignment Operator divides a variable by the value of the right operand and assigns the remainder to the variable.
Exponentiation Assignment Operator
The Exponentiation Assignment Operator raises the value of a variable to the power of the right operand.
Left Shift Assignment Operator(<<=)
This Left Shift Assignment O perator moves the specified amount of bits to the left and assigns the result to the variable.
Right Shift Assignment O perator(>>=)
The Right Shift Assignment Operator moves the specified amount of bits to the right and assigns the result to the variable.
Bitwise AND Assignment Operator(&=)
The Bitwise AND Assignment Operator uses the binary representation of both operands, does a bitwise AND operation on them, and assigns the result to the variable.
Btwise OR Assignment Operator(|=)
The Btwise OR Assignment Operator uses the binary representation of both operands, does a bitwise OR operation on them, and assigns the result to the variable.
Bitwise XOR Assignment Operator(^=)
The Bitwise XOR Assignment Operator uses the binary representation of both operands, does a bitwise XOR operation on them, and assigns the result to the variable.
Logical AND Assignment Operator(&&=)
The Logical AND Assignment assigns the value of y into x only if x is a truthy value.
Logical OR Assignment Operator( ||= )
The Logical OR Assignment Operator is used to assign the value of y to x if the value of x is falsy.
Nullish coalescing Assignment Operator(??=)
The Nullish coalescing Assignment Operator assigns the value of y to x if the value of x is null.
Supported Browsers: The browsers supported by all JavaScript Assignment operators are listed below:
- Google Chrome
- Microsoft Edge
JavaScript Assignment Operators – FAQs
What are assignment operators in javascript.
Assignment operators in JavaScript are used to assign values to variables. The most common assignment operator is the equals sign (=), but there are several other assignment operators that perform an operation and assign the result to a variable in a single step.
What is the basic assignment operator?
The basic assignment operator is =. It assigns the value on the right to the variable on the left.
What are compound assignment operators?
Compound assignment operators combine a basic arithmetic or bitwise operation with assignment. For example, += combines addition and assignment.
What does the += operator do?
The += operator adds the value on the right to the variable on the left and then assigns the result to the variable.
How does the *= operator work?
The *= operator multiplies the variable by the value on the right and assigns the result to the variable.
Can you use assignment operators with strings?
Yes, you can use the += operator to concatenate strings.
What is the difference between = and ==?
The = operator is the assignment operator, used to assign a value to a variable. The == operator is the equality operator, used to compare two values for equality, performing type conversion if necessary.
What does the **= operator do?
The **= operator performs exponentiation (raising to a power) and assigns the result to the variable.
Please Login to comment...
Similar reads.
- Web Technologies
- javascript-operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
JS Reference
Html events, html objects, other references, javascript operators reference, javascript operators.
Operators are used to assign values, compare values, perform arithmetic operations, and more.
There are different types of JavaScript operators:
- Arithmetic Operators
- Assignment Operators
Comparison Operators
Logical operators.
- Conditional Operators
- Type Operators
JavaScript Arithmetic Operators
Arithmetic operators are used to perform arithmetic between variables and/or values.
Given that y = 5 , the table below explains the arithmetic operators:
Oper | Name | Example | Results | Try it |
---|---|---|---|---|
+ | Addition | x = y + 2 | y=5, x=7 | |
- | Subtraction | x=y-2 | y=5, x=3 | |
* | Multiplication | x=y*2 | y=5, x=10 | |
** | Exponentiation | x=y**2 | y=5, x=25 | |
/ | Division | x = y / 2 | y=5, x=2.5 | |
% | Remainder | x = y % 2 | y=5, x=1 | |
++ | Pre increment | x = ++y | y=6, x=6 | |
++ | Post increment | x = y++ | y=6, x=5 | |
-- | Pre decrement | x = --y | y=4, x=4 | |
-- | Post decrement | x = y-- | y=4, x=5 |
JavaScript Assignment Operators
Assignment operators are used to assign values to JavaScript variables.
Given that x = 10 and y = 5 , the table below explains the assignment operators:
Oper | Example | Same As | Result | Try it |
---|---|---|---|---|
= | x = y | x = y | x = 5 | |
+= | x += y | x = x + y | x = 15 | |
-= | x -= y | x = x - y | x = 5 | |
*= | x *= y | x = x * y | x = 50 | |
/= | x /= y | x = x / y | x = 2 | |
%= | x %= y | x = x % y | x = 0 | |
: | x: 45 | size.x = 45 | x = 45 |
Advertisement
JavaScript String Operators
The + operator, and the += operator can also be used to concatenate (add) strings.
Given that t1 = "Good " , t2 = "Morning" , and t3 = "" , the table below explains the operators:
Oper | Example | t1 | t2 | t3 | Try it |
---|---|---|---|---|---|
+ | t3 = t1 + t2 | "Good " | "Morning" | "Good Morning" | |
+= | t1 += t2 | "Good Morning" | "Morning" |
Comparison operators are used in logical statements to determine equality or difference between variables or values.
Given that x = 5 , the table below explains the comparison operators:
Oper | Name | Comparing | Returns | Try it |
---|---|---|---|---|
== | equal to | x == 8 | false | |
== | equal to | x == 5 | true | |
=== | equal value and type | x === "5" | false | |
=== | equal value and type | x === 5 | true | |
!= | not equal | x != 8 | true | |
!== | not equal value or type | x !== "5" | true | |
!== | not equal value or type | x !== 5 | false | |
> | greater than | x > 8 | false | |
< | less than | x < 8 | true | |
>= | greater or equal to | x >= 8 | false | |
<= | less or equal to | x <= 8 |
Conditional (Ternary) Operator
The conditional operator assigns a value to a variable based on a condition.
Syntax | Example | Try it |
---|---|---|
(condition) ? x : y | (z < 18) ? x : y |
Logical operators are used to determine the logic between variables or values.
Given that x = 6 and y = 3 , the table below explains the logical operators:
Oper | Name | Example | Try it |
---|---|---|---|
&& | AND | (x < 10 && y > 1) is true | |
|| | OR | (x === 5 || y === 5) is false | |
! | NOT | !(x === y) is true |
The Nullish Coalescing Operator (??)
The ?? operator returns the first argument if it is not nullish ( null or undefined ).
Otherwise it returns the second argument.
The nullish operator is supported in all browsers since March 2020:
Chrome 80 | Edge 80 | Firefox 72 | Safari 13.1 | Opera 67 |
Feb 2020 | Feb 2020 | Jan 2020 | Mar 2020 | Mar 2020 |
The Optional Chaining Operator (?.)
The ?. operator returns undefined if an object is undefined or null (instead of throwing an error).
The optional chaining operator is supported in all browsers since March 2020:
JavaScript Bitwise Operators
Bit operators work on 32 bits numbers. Any numeric operand in the operation is converted into a 32 bit number. The result is converted back to a JavaScript number.
Oper | Name | Example | Same as | Result | Decimal | Try it |
---|---|---|---|---|---|---|
& | AND | x = 5 & 1 | 0101 & 0001 | 0001 | 1 | |
| | OR | x = 5 | 1 | 0101 | 0001 | 0101 | 5 | |
~ | NOT | x = ~ 5 | ~0101 | 1010 | 10 | |
^ | XOR | x = 5 ^ 1 | 0101 ^ 0001 | 0100 | 4 | |
<< | Left shift | x = 5 << 1 | 0101 << 1 | 1010 | 10 | |
>> | Right shift | x = 5 >> 1 | 0101 >> 1 | 0010 | 2 | |
>>> | Unsigned right | x = 5 >>> 1 | 0101 >>> 1 | 0010 | 2 |
The table above uses 4 bits unsigned number. Since JavaScript uses 32-bit signed numbers, ~ 5 will not return 10. It will return -6. ~00000000000000000000000000000101 (~5) will return 11111111111111111111111111111010 (-6)
The typeof Operator
The typeof operator returns the type of a variable, object, function or expression:
Please observe:
- The data type of NaN is number
- The data type of an array is object
- The data type of a date is object
- The data type of null is object
- The data type of an undefined variable is undefined
You cannot use typeof to define if a JavaScript object is an array or a date.
Both array and date return object as type.
The delete Operator
The delete operator deletes a property from an object:
The delete operator deletes both the value of the property and the property itself.
After deletion, the property cannot be used before it is added back again.
The delete operator is designed to be used on object properties. It has no effect on variables or functions.
The delete operator should not be used on the properties of any predefined JavaScript objects (Array, Boolean, Date, Function, Math, Number, RegExp, and String).
This can crash your application.
The Spread (...) Operator
The ... operator can be used to expand an iterable into more arguments for function calls:
The in Operator
The in operator returns true if a property is in an object, otherwise false:
Object Example
You cannot use in to check for array content like ("Volvo" in cars).
Array properties can only be index (0,1,2,3...) and length.
See the examples below.
Predefined Objects
The instanceof operator.
The instanceof operator returns true if an object is an instance of a specified object:
The void Operator
The void operator evaluates an expression and returns undefined . This operator is often used to obtain the undefined primitive value, using "void(0)" (useful when evaluating an expression without using the return value).
JavaScript Operator Precedence

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Skip to main content
- Select language
- Skip to search
- Assignment operators
An assignment operator assigns a value to its left operand based on the value of its right operand.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Name | Shorthand operator | Meaning |
---|---|---|
Simple assignment operator which assigns a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
This is an experimental technology, part of the ECMAScript 2016 (ES7) proposal. Because this technology's specification has not stabilized, check the compatibility table for usage in various browsers. Also note that the syntax and behavior of an experimental technology is subject to change in future version of browsers as the spec changes.
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.
Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.
Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
Specifications
Specification | Status | Comment |
---|---|---|
Draft | ||
Standard | ||
Standard | ||
Standard | Initial definition. |
Browser compatibility
Feature | Chrome | Edge | Firefox (Gecko) | Internet Explorer | Opera | Safari |
---|---|---|---|---|---|---|
Basic support | (Yes) | (Yes) | (Yes) | (Yes) | (Yes) | (Yes) |
Feature | Android | Chrome for Android | Edge | Firefox Mobile (Gecko) | IE Mobile | Opera Mobile | Safari Mobile |
---|---|---|---|---|---|---|---|
Basic support | (Yes) | (Yes) | (Yes) | (Yes) | (Yes) | (Yes) | (Yes) |
- Arithmetic operators
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project

JAVASCRIPT ASSIGNMENT OPERATORS
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript.
Assignment Operators
In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
The assignment operators are used to assign value based on the right operand to its left operand.
The left operand must be a variable while the right operand may be a variable, number, boolean, string, expression, object, or combination of any other.
One of the most basic assignment operators is equal = , which is used to directly assign a value.
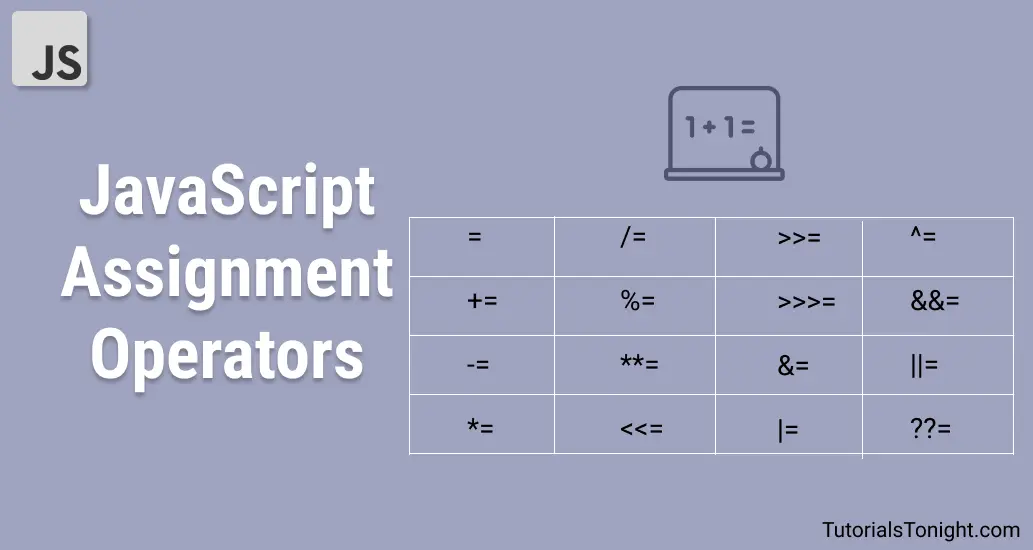
Assignment Operators List
Here is the list of all assignment operators in JavaScript:
In the following table if variable a is not defined then assume it to be 10.
Operator | Description | Example | Equivalent to |
---|---|---|---|
= | a = 10 | a = 10 | |
+= | a += 10 | a = a + 10 | |
-= | a -= 10 | a = a - 10 | |
*= | a *= 10 | a = a * 10 | |
/= | a /= 10 | a = a / 10 | |
%= | a %= 10 | a = a % 10 | |
**= | a **= 2 | a = a ** 2 | |
<<= | a <<= 1 | a = a << 1 | |
>>= | a >>= 2 | a = a >> 2 | |
>>>= | a >>>= 1 | a = a >>> 1 | |
&= | a &= 4 | a = a & 4 | |
|= | a |= 2 | a = a | 2 | |
^= | a ^= 5 | a = a ^ 5 | |
&&= | a &&= 3 | a = a && 3 | |
||= | a ||= 4 | a = a || 4 | |
??= | a ??= 2 | a = a ?? 2 |
Assignment operator
The assignment operator = is the simplest value assigning operator which assigns a given value to a variable.
The assignment operators support chaining, which means you can assign a single value in multiple variables in a single line.
Addition assignment operator
The addition assignment operator += is used to add the value of the right operand to the value of the left operand and assigns the result to the left operand.
On the basis of the data type of variable, the addition assignment operator may add or concatenate the variables.
Subtraction assignment operator
The subtraction assignment operator -= subtracts the value of the right operand from the value of the left operand and assigns the result to the left operand.
If the value can not be subtracted then it results in a NaN .
Multiplication assignment operator
The multiplication assignment operator *= assigns the result to the left operand after multiplying values of the left and right operand.
Division assignment operator
The division assignment operator /= divides the value of the left operand by the value of the right operand and assigns the result to the left operand.
Remainder assignment operator
The remainder assignment operator %= assigns the remainder to the left operand after dividing the value of the left operand by the value of the right operand.
Exponentiation assignment operator
The exponential assignment operator **= assigns the result of exponentiation to the left operand after exponentiating the value of the left operand by the value of the right operand.
Left shift assignment
The left shift assignment operator <<= assigns the result of the left shift to the left operand after shifting the value of the left operand by the value of the right operand.
Right shift assignment
The right shift assignment operator >>= assigns the result of the right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Unsigned right shift assignment
The unsigned right shift assignment operator >>>= assigns the result of the unsigned right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Bitwise AND assignment
The bitwise AND assignment operator &= assigns the result of bitwise AND to the left operand after ANDing the value of the left operand by the value of the right operand.
Bitwise OR assignment
The bitwise OR assignment operator |= assigns the result of bitwise OR to the left operand after ORing the value of left operand by the value of the right operand.
Bitwise XOR assignment
The bitwise XOR assignment operator ^= assigns the result of bitwise XOR to the left operand after XORing the value of the left operand by the value of the right operand.
Logical AND assignment
The logical AND assignment operator &&= assigns value to left operand only when it is truthy .
Note : A truthy value is a value that is considered true when encountered in a boolean context.
Logical OR assignment
The logical OR assignment operator ||= assigns value to left operand only when it is falsy .
Note : A falsy value is a value that is considered false when encountered in a boolean context.
Logical nullish assignment
The logical nullish assignment operator ??= assigns value to left operand only when it is nullish ( null or undefined ).
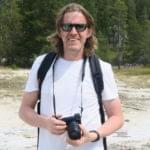
Demystifying JavaScript Operators: What Does That Symbol Mean?
Share this article
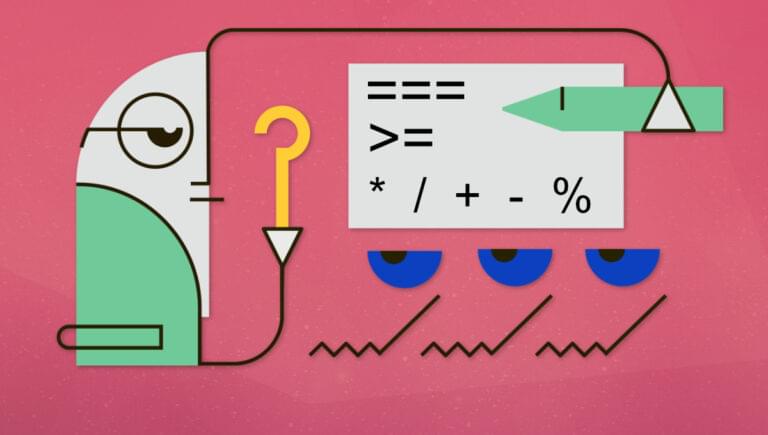
What are JavaScript Operators?
A quick word on terminology, arithmetic operators, assignment operators, comparison operators, logical operators, bitwise operators, other operators, frequently asked questions (faqs) about javascript operators.
In this article, we’re going to examine the operators in JavaScript one by one. We’ll explain their function and demonstrate their usage, helping you to grasp their role in building more complex expressions.
JavaScript, a cornerstone of modern web development, is a robust language full of numerous features and constructs. Among these, operators (special symbols such as + , += , && , or ... ) play an essential role, enabling us to perform different types of calculations and manipulations on variables and values.
Despite their importance, operators can sometimes be a source of confusion for new programmers and seasoned coders alike.
Take a moment to examine this code snippet:
Don’t be alarmed if it seems a bit cryptic. By the time we’re finished, you’ll be able to understand exactly what it does.
Before we dive in, let’s clarify a couple of terms that we’ll be using quite a bit:
An operand is the item that operators work on. If we think of an operator as a kind of action, the operand is what the action is applied to. For example, in the expression 5 + 3, + is the operator (the action of addition), and 5 and 3 are the operands — the numbers being added together. In JavaScript, operands can be of various types, such as numbers, strings, variables, or even more complex expressions.
Coercion is the process of converting a value from one primitive type to another. For example, JavaScript might change a number into a string, or a non-Boolean value into a Boolean. The primitive types in JavaScript are String , Number , BigInt , Boolean , undefined , Symbol or null .
NaN stands for Not a Number . It’s a special value of the Number type that represents an invalid or unrepresentable numeric value.
Truthy values are those that evaluate to true in a Boolean context, while falsy values evaluate to false — with falsy values being false , 0 , -0 , '' , null , undefined , NaN and BigInt(0) . You can read more about truthy and falsy values in Truthy and Falsy Values: When All is Not Equal in JavaScript .
As we explore JavaScript operators, we’ll see these concepts in action and get a better understanding of how they influence the results of our operations.
Arithmetic operators allow us to perform arithmetic operations on values and to transform data. The commonly used arithmetic operators in JavaScript include addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). Beyond these, we also have the modulus operator ( % ), which returns the remainder of a division operation, and the increment ( ++ ) and decrement ( -- ) operators that modify a value by one.
Addition: +
The addition operator performs two distinct operations: numeric addition and string concatenation. When evaluating expressions using the + operator, JavaScript first coerces both operands to primitive values. Once this is done, it examines the types of both operands.
If one operand is a string, the other operand is also converted to a string, and then the two strings are concatenated. For example:
If both operands are BigInts , BigInt addition is performed. A BigInt is a special numeric type that can deal with numbers larger than the standard Number type can handle.
But if one operand is a BigInt and the other isn’t, JavaScript throws a TypeError :
For all other cases, both operands are converted to numbers, and numeric addition is performed. For example:
Be aware that JavaScript sometimes has a strange idea of what this looks like:
In this case, JavaScript tried to convert the object { a: 1 } to a primitive value, but the best it could do was to convert it to the string [object Object] , which then got concatenated with the number 1.
Subtraction: -
The subtraction operator in JavaScript is straightforward in its usage: it’s used to subtract one number from another. Like the addition operator, it also works with the BigInt type.
If both operands are numbers or can be converted to numbers, JavaScript performs numeric subtraction:
If both operands are BigInts , JavaScript performs BigInt subtraction:
Like the addition operator, subtraction can also produce unexpected results when used with non-numbers. For example, if we try to subtract a something that can’t be converted to a number, JavaScript will return NaN , which stands for “Not a Number”:
Multiplication: *
The multiplication operator works with numbers and BigInts.
Normally, we’ll be multiplying numbers:
If both operands are BigInts , then it performs BigInt multiplication:
As with other operators, JavaScript attempts to convert non-numeric values into numbers. If it can’t do this, it returns NaN :
Division: /
The division operator ( / ) functions with numbers and BigInts , much the same way as + , - and * . It first converts both operands into numbers.
Standard number division:
When dealing with BigInts , the division operator behaves slightly differently. It performs the division and discards any remainder, effectively truncating the result towards zero:
Dividing a number by zero will produce Infinity , unless it’s a BigInt , in which case it throws a RangeError .
If we attempt to divide a number by a value that can’t be converted into a number, JavaScript will usually return NaN .
Modulus (remainder): %
The modulus operator is used to find the remainder after division of one number by another (known as the dividend and the divisor ). This arithmetic operator works with numbers and BigInts .
When we use the % operator, JavaScript first converts the operands to numbers:
This is because three goes into ten three times (making nine), and what’s left over (the remainder) is one.
A common use case for this operator is to check if a number is odd or even:
This uses an arrow function and the triple equals operator that we’ll meet later on.
The modulus operator has some special cases. For example, if one of the operands is NaN , if the dividend is Infinity , or if the divisor is 0 , the operation returns NaN .
On the other hand, if the divisor is Infinity or if the dividend is 0 , the operation returns the dividend.
Increment: ++
The increment operator is used to increase the value of a variable by 1. It can be applied to operands of type Number or BigInt , and its behavior can differ based on whether it’s used in postfix or prefix form.
Postfix increment
If the operator is placed after the operand ( num++ ), the increment operation is performed after the value is returned. In this case, the original value of the variable is used in the current expression, and the variable’s value is incremented afterward:
Prefix increment
If the operator is placed before the operand ( ++num ), the increment operation is performed before the value is returned. In this case, the variable’s value is incremented first, and then the updated value is used in the current expression:
Decrement: --
The decrement operator is used to decrease the value of a variable by 1. Similar to the increment operator, it can be applied to operands of type Number or BigInt . The behavior of the decrement operator can vary based on whether it’s used in postfix or prefix form.
Postfix decrement
When the operator is placed after the operand ( num-- ), the decrement operation is performed after the value is returned. In this case, the original value of the variable is used in the current expression, and the variable’s value is decremented afterward:
Prefix decrement
When the operator is placed before the operand ( --num ), the decrement operation is performed before the value is returned. In this case, the variable’s value is decremented first, and then the updated value is used in the current expression:
The decrement operator, just like the increment operator, can only be used with variables that can be changed:
Miscellaneous arithmetic operators
In addition to the increment and decrement operators, there are other arithmetic operators in JavaScript that we should be aware of.
The unary negation operator ( - ) is used to negate a numeric value, changing its sign to the opposite. For example, -5 would be the negation of the number 5 .
The unary plus operator ( + ) can be used to explicitly convert a value to a number, which can be useful when dealing with string representations of numbers. For example, +'10' converts the string '10' to the number 10 :
The exponentiation operator ( ** ) is used to raise a number to a power. For example, 2 ** 3 represents 2 raised to the power of 3, which results in 8.
It’s also important to note that JavaScript follows operator precedence rules, which determine the order in which operators are evaluated in an expression. For example, multiplication and division have a higher precedence than addition and subtraction, so they are evaluated first:
We can alter the order of evaluation by using the grouping operator () , which is covered in the “ Grouping operator ” section below.
Assignment operators are used to assign values to variables. They also offer a concise and effective way to update the value of a variable based on an expression or other value. In addition to the basic assignment operator ( = ), JavaScript provides compound assignment operators that combine arithmetic or logical operations with assignment.
Assignment: =
This operator is used to assign a value to a variable. It allows us to store a value in a variable so that we can use and reference it later in our code:
The assignment operator assigns the value on the right-hand side of the operator to the variable on the left-hand side.
Additionally, the = operator can be chained to assign the same value to multiple variables in a single line:
Addition assignment: +=
The addition assignment operator is a compound operator that performs an operation and assignment in one step. Specifically, it adds the right operand to the left operand and then assigns the result to the left operand:
This operator isn’t limited to numbers. It can also be used for string concatenation:
When the operands aren’t of the same type, JavaScript applies the same rules of type coercion that we saw previously:
Subtraction assignment: -=
The subtraction assignment operator is another compound operator that performs an operation and assignment in one step. It subtracts the right operand from the left operand and then assigns the result to the left operand:
Like other JavaScript operators, -= performs type coercion when the operands aren’t of the same type. If an operand can be converted to a number, JavaScript will do so:
Otherwise, the result is NaN :
Multiplication assignment: *=
The multiplication assignment operator multiplies the left operand by the right operand and then assigns the result back to the left operand:
When we use operands of different types, JavaScript will try to convert non-numeric string operands to numbers:
If the string operand can’t be converted to a number, the result is NaN .
Division assignment: /=
Like its siblings, the division assignment operator performs an operation on the two operands and then assigns the result back to the left operand:
Otherwise, the rules we discussed for the division operator above apply:
Modulus assignment: %=
The modulus assignment operator performs a modulus operation on the two operands and assigns the result to the left operand:
Otherwise, the rules we discussed for the modulus operator apply:
Exponentiation assignment: **=
The exponentiation assignment operator performs exponentiation, where the left operand is the base and the right operand is the exponent, and assigns the result to the left operand:
Here, num is raised to the power of 3, and the result (8) is assigned back to num .
As before, when the second operand is not a number, JavaScript will attempt to convert it with varying degrees of success:

Bitwise assignment operators
While we’ve been focusing on arithmetic operators so far, JavaScript also supports a set of assignment operators that work at the bit level. These are the bitwise assignment operators . If you’re familiar with binary numbers and bit manipulation, these operators will be right up your alley.
These operators include:
- Bitwise AND assignment ( &= )
- Bitwise OR assignment ( |= )
- Bitwise XOR assignment ( ^= )
- Left shift assignment ( <<= )
- Right shift assignment ( >>= )
- Unsigned right shift assignment ( >>>= )
Each of these JavaScript assignment operators performs a specific bitwise operation on the binary representations of the numbers involved and assigns the result back to the left operand.
We’ll explore bitwise operators in more detail in the “ Bitwise Operators ” section below.
Leaving the realm of arithmetic, let’s dive into another significant group of JavaScript operators — the comparison operators. As the name implies, comparison operators are used to compare values and return a Boolean result. Comparison operators are the underpinning of many programming decisions and control structures — from simple condition checks to complex logical operations.
Equality: ==
The equality operator is used to check whether two values are equal to each other. It returns a Boolean result. However, it’s important to note that this comparison operator performs a loose equality check, meaning that if the operands are of different types, JavaScript will try to convert them to a common type before making the comparison:
Things get slightly more complicated dealing with objects and arrays. The == operator checks whether they refer to the same location in memory, not whether their contents are identical:
In this case, JavaScript doesn’t attempt to convert and compare the values within the objects or arrays. Instead, it checks whether they’re the same object (that is, whether they occupy the same memory space).
You can read more about loose equality comparisons here .
Inequality: !=
The inequality operator is used to check whether two values are not equal to each other. Like the == operator, it performs a loose inequality check. This means that, if they’re of different types, it will try to convert the operands to a common type before making the comparison:
Similar to the == operator, when comparing objects and arrays, the != operator checks whether they refer to the same memory location, not whether their content is identical.
Strict equality ( === ) and strict inequality ( !== )
The strict equality and strict inequality comparison operators are similar to their non-strict counterparts ( == and != ), but they don’t perform type coercion. If the operands are of different types, they’re considered different, no matter their values.
Here’s how they work:
For objects and arrays, the strict equality operator behaves the same way as the loose equality operator: it checks whether they refer to the same object, not whether their contents are identical.
NaN is a special case. It’s the only value in JavaScript that isn’t strictly equal to itself:
You can read more about strict equality comparisons here .
Greater than: >
The greater than operator checks if the left operand is greater than the right operand, returning a Boolean result. This comparison is straightforward with numeric values:
When comparing non-numeric values, JavaScript applies a process of conversion to make them comparable. If both values are strings, they’re compared based on their corresponding positions in the Unicode character set:
If one or both of the operands aren’t strings, JavaScript tries to convert them to numeric values for the comparison:
Certain special rules apply for specific values during this conversion. For instance, null is converted to 0 , undefined is converted to NaN , and Boolean values true and false are converted to 1 and 0 respectively. However, if either value is NaN after conversion, the operator will always return false :
Less than: <
The less than operator returns true if the left operand is less than the right operand, and false otherwise. The same rules apply as for the greater than operator; only the order of operands is reversed:
Like the > operator, the < operator uses coercion to convert operands to a common type before making the comparison.
Greater than or equal to ( >= ) and less than or equal to ( <= )
The greater than or equal to ( >= ) and less than or equal to ( <= ) operators function similarly to their < and > counterparts, with the added condition of equality.
For the >= operator, it returns true if the left operand is greater than or equal to the right operand, and false otherwise. Conversely, the <= operator returns true if the left operand is less than or equal to the right operand, and false otherwise. Coercion rules and type conversion, as explained in the sections on < and > directly above, apply here as well:
Logical operators in JavaScript offer a way to work with multiple conditions simultaneously. They’re an integral part of decision-making constructs in programming, such as if statements, and for loops.
Mastering logical operators is key for controlling the flow of our code.
Logical AND: &&
When used with Boolean values, the logical AND operator returns true if all conditions are true and false otherwise.
However, with non-Boolean values, it gets more interesting:
- The operator evaluates conditions from left to right.
- If it encounters a value that can be converted to false (known as a falsy value), it stops and returns that value.
- If all values are truthy, it returns the last truthy value.
For example:
The && operator’s ability to return the value of the operands makes it a versatile tool for conditional execution and setting default values. For example, we can use the && operator to execute a function or a block of code only if a certain condition is met:
In this case, renderWelcomeMessage will only be executed if userIsLoggedIn is true . If userIsLoggedIn is false , the operation will stop at userIsLoggedIn and renderWelcomeMessage won’t be called. This pattern is often used with React to conditionally render components.
Logical OR: ||
When used with Boolean values, the logical OR operator returns true if at least one condition is true and false otherwise.
It can also return non-Boolean values, performing what’s known as short-circuit evaluation . It evaluates conditions from left to right, stopping and returning the value of the first truthy condition encountered. If all conditions are falsy, it returns the last falsy value:
Logical NOT: !
The logical NOT operator is used to reverse the Boolean value of a condition or expression. Unlike the && and || operators, the ! operator always returns a Boolean value.
If the condition is truthy (that is, it can be converted to true ), the operator returns false . If the condition is falsy (that is, it can be converted to false ), the operator returns true :
We can use the ! operator twice to convert a value to its Boolean equivalent:
Nullish coalescing operator: ??
The nullish coalescing operator checks whether the value on its left is null or undefined , and if so, it returns the value on the right. Otherwise, it returns the value on the left.
Though similar to the || operator in some respects, the ?? operator differs in its handling of falsy values.
Whereas the || operator returns the right-hand operand if the left-hand operand is any falsy value (such as null , undefined , false , 0 , NaN , '' ), the ?? operator only does so when the left-hand operand is null or undefined :
Setting default values with logical operators
The || and ?? logical operators are useful for setting default values in programs. Here’s an example of doing this with the || operator:
Here’s an example with the ?? operator:
The main difference between these logical operators (as highlighted above) is how they treat falsy values:
Bitwise operators in JavaScript offer a way to perform operations at the binary level, directly manipulating bits in a number’s binary representation. While these operators can be instrumental in specific tasks like data encoding, decoding, and processing, they’re not frequently used in day-to-day JavaScript programming.
In this article, we’ll provide an overview of these operators so you can recognize and understand them, but we won’t delve deeply into their usage given their relatively niche application.
Bitwise AND: &
The bitwise AND operator performs a bitwise AND operation on the binary representations of integers. It returns a new number whose bits are set to 1 if the bits in the same position in both operands are 1. Otherwise, it sets them to 0:
Bitwise OR: |
The bitwise OR operator works similarly to the & operator, but it sets a bit to 1 if at least one of the bits in the same position in the operands is 1:
Bitwise XOR: ^
The bitwise XOR operator is a little different. It sets a bit to 1 only if the bits in the same position in the operands are different (one is 1 and the other is 0):
Bitwise NOT: ~
The bitwise NOT operator ( ~ ) inverts the bits of its operand. It switches 1s to 0s and 0s to 1s in the binary representation of a number:
Note: two’s complement is a method for representing negative integers in binary notation.
Bitwise shift operators: <<, >>, >>>
Bitwise shift operators are used to shift the bits of a binary number to the left or right. In JavaScript, there are three types: left shift ( << ), right shift ( >> ), and zero-fill right shift ( >>> ).
The left shift bitwise operator ( << ) moves bits to the left and fills in with zeros on the right. The right shift operator ( >> ) shifts bits to the right, discarding bits shifted off. The zero-fill right shift operator ( >>> ) also shifts bits to the right but fills in zeros on the left.
These operators are less common in everyday JavaScript coding, but they have uses in more specialized areas like low-level programming, binary data manipulation, and some types of mathematical calculations.
Apart from the commonly used arithmetic, comparison, logical, and bitwise operators, JavaScript offers a variety of unique operators for specific purposes. These include operators for handling conditional logic, managing object properties, controlling the order of operations, and more.
Conditional (ternary) operator: ? :
The conditional ternary operator ( ? : ) is a concise way to make decisions in our JavaScript code. It gets its name from being the only operator that takes three operands. The syntax for this conditional operator is as follows:
The operator works by first evaluating the condition. If the condition is true , it executes the expressionIfTrue , and if it’s false , it executes the expressionIfFalse :
In the code above, the ternary operator checks if age is greater than or equal to 18. Since age is 15, the condition evaluates to false , and 'Minor' is assigned to the status variable.
This operator can be a handy way to write concise if–else statements, but it can also make code more difficult to read if overused or used in complex conditions.
Spread operator: ...
The spread operator ( ... ) allows elements of an iterable (such as an array or a string) to be expanded in places where zero or more arguments or elements are expected. It can be used in function calls, array literals, and object literals:
The spread operator can be a handy tool for creating copies of arrays or objects, concatenating arrays, or passing the elements of an array as arguments to a function.
You can read more about the spread operator in Quick Tip: How to Use the Spread Operator in JavaScript .
Comma operator: ,
The comma operator ( , ) allows multiple expressions to be evaluated in a sequence and returns the result of the last expression. The expressions are evaluated from left to right.
It’s particularly useful when we need to include multiple expressions in a location that only allows for one, such as in the initialization or update sections of a for loop:
In the code above, the comma operator is used to declare and update two variables ( i and j ) in the for loop.
Optional chaining operator: ?.
Optional chaining is a relatively recent addition to JavaScript (as of ES2020) that simplifies the process of accessing deeply nested properties of objects. It helps us to write cleaner and safer code by providing a way to attempt to retrieve a property value without having to explicitly check if each reference in its chain exists:
In the above example, user?.address?.city accesses the city property of user.address if user and user.address both exist. Otherwise, it returns undefined . This approach avoids a common pitfall in JavaScript, where trying to access a property of undefined or null leads to a TypeError :
Before optional chaining, JavaScript developers had to use lengthy conditional logic to avoid such errors:
Pipeline operator: |>
The pipeline operator ( |> ) is intended to improve the readability of code that would otherwise be written with nested function calls. Essentially, it allows us to take the result of one expression and feed it into the next. This is particularly useful when we’re applying a series of transformations to a value:
With the pipeline operator, we’re able rewrite this code like so:
Be aware that, at the time of writing, the pipeline operator is at stage 2 of the ECMAScript proposal process , meaning it’s a draft and is undergoing further iteration and refinement.
Grouping operator: ()
The grouping operator ( () ) in JavaScript is used to change the precedence of evaluation in expressions. This operator doesn’t perform any operations on its value, but it controls the order in which calculations are carried out within an expression.
For example, multiplication and division have higher precedence than addition and subtraction. This means that, in an expression such as 2 + 3 * 4 , the multiplication is done first, resulting in 2 + 12 , and then the addition is performed, giving a result of 14 .
If we want to change the order of operations, we can use the grouping operator. For example, if we want the addition to be done before the multiplication in the previous example, we could write (2 + 3) * 4 . In this case, the addition is performed first, resulting in 5 * 4 , and then the multiplication is performed, giving a result of 20.
The grouping operator allows us to ensure that operations are performed in the order we intend, which can be critical in more complex mathematical or logical expressions.
We’ve spent this article delving into the broad and sometimes complex world of JavaScript operators. These operators enable us to manipulate data, control program flow, and carry out complex computations.
Understanding these operators is not a mere academic exercise; it’s a practical skill that can enhance our ability to write and understand JavaScript.
Remember the confusing code snippet we started with? Let’s revisit that and see if it makes more sense now:
In plain English, this code is finding the maximum of three numbers, x , y , and z .
It does this by using a combination of JavaScript’s ternary operator ( ? : ) and comparison operators ( > ).
Here’s how it works:
- The expression (x > y ? x : y) checks whether x is greater than y .
- If x is greater than y , it returns x ; otherwise, it returns y . In other words, it’s getting the maximum of x and y .
- This result (the maximum of x and y ) is then compared to z with the > operator.
- If the maximum of x and y is greater than z , then second ternary (x > y ? x : y) is evaluated.
- In this ternary, x and y are compared once again and the greater of the two is returned.
- Otherwise, if z is greater than the maximum of x and y , then z is the maximum of the three numbers, and it’s returned.
If you found this explanation confusing, that’s because it is. Nesting ternary operators like this isn’t very readable and the calculation could be better expressed using Math.max :
Nonetheless, understanding how JavaScript operators work is like learning the grammar of a new language. It can be challenging at first, but once we’ve grasped the basics, we’ll be constructing complex sentences (or in our case, code) with ease.
Before I go, if you found this guide helpful and are looking to dive deeper into JavaScript, why not check out Learn to Code with JavaScript over on SitePoint Premium. This book is an ideal starting point for beginners, teaching not just JavaScript — the world’s most popular programming language — but also essential coding techniques that can be applied to other programming languages.
It’s a fun and easy-to-follow guide that will turn you from a novice to a confident coder in no time.
Happy coding!
What are the different types of JavaScript operators and how are they used?
JavaScript operators are symbols that are used to perform operations on operands (values or variables). There are several types of operators in JavaScript, including arithmetic, assignment, comparison, logical, bitwise, string, ternary, and special operators. Each type of operator has a specific function. For example, arithmetic operators are used to perform mathematical operations, while assignment operators are used to assign values to variables. Comparison operators are used to compare two values, and logical operators are used to determine the logic between variables or values.
How does the assignment operator work in JavaScript?
The assignment operator in JavaScript is used to assign values to variables. It is represented by the “=” symbol. For example, if you have a variable named “x” and you want to assign the value “10” to it, you would write “x = 10”. This means that “x” now holds the value “10”. There are also compound assignment operators that perform an operation and an assignment in one step. For example, “x += 5” is the same as “x = x + 5”.
What is the difference between “==” and “===” in JavaScript?
In JavaScript, “==” and “===” are comparison operators, but they work slightly differently. The “==” operator compares the values of two operands for equality, after converting both operands to a common type. On the other hand, the “===” operator, also known as the strict equality operator, compares both the value and the type of the operands. This means that “===” will return false if the operands are of different types, even if their values are the same.
How do logical operators work in JavaScript?
Logical operators in JavaScript are used to determine the logic between variables or values. There are three logical operators: AND (&&), OR (||), and NOT (!). The AND operator returns true if both operands are true, the OR operator returns true if at least one of the operands is true, and the NOT operator returns the opposite of the operand.
What is the purpose of the ternary operator in JavaScript?
The ternary operator in JavaScript is a shorthand way of writing an if-else statement. It is called the ternary operator because it takes three operands: a condition, a value to return if the condition is true, and a value to return if the condition is false. The syntax is “condition ? value_if_true : value_if_false”. For example, “x = (y > 5) ? 10 : 20” means that if “y” is greater than 5, “x” will be assigned the value 10; otherwise, “x” will be assigned the value 20.
How are bitwise operators used in JavaScript?
Bitwise operators in JavaScript operate on 32-bit binary representations of the number values. They perform bit-by-bit operations on these binary representations. There are several bitwise operators, including AND (&), OR (|), XOR (^), NOT (~), left shift (<<), right shift (>>), and zero-fill right shift (>>>). These operators can be used for tasks such as manipulating individual bits in a number, which can be useful in certain types of programming, such as graphics or cryptography.
What are string operators in JavaScript?
In JavaScript, the plus (+) operator can be used as a string operator. When used with strings, the + operator concatenates (joins together) the strings. For example, “Hello” + ” World” would result in “Hello World”. There is also a compound assignment string operator (+=) that can be used to add a string to an existing string. For example, “text += ‘ World'” would add ” World” to the end of the existing string stored in the “text” variable.
What are special operators in JavaScript?
Special operators in JavaScript include the conditional (ternary) operator, the comma operator, the delete operator, the typeof operator, the void operator, and the in operator. These operators have specific uses. For example, the typeof operator returns a string indicating the type of a variable or value, and the delete operator deletes a property from an object.
How does operator precedence work in JavaScript?
Operator precedence in JavaScript determines the order in which operations are performed when an expression involves multiple operators. Operators with higher precedence are performed before those with lower precedence. For example, in the expression “2 + 3 * 4”, the multiplication is performed first because it has higher precedence than addition, so the result is 14, not 20.
Can you explain how increment and decrement operators work in JavaScript?
Increment (++) and decrement (–) operators in JavaScript are used to increase or decrease a variable’s value by one, respectively. They can be used in a prefix form (++x or –x) or a postfix form (x++ or x–). In the prefix form, the operation is performed before the value is returned, while in the postfix form, the operation is performed after the value is returned. For example, if x is 5, ++x will return 6, but x++ will return 5 (although x will be 6 the next time it is accessed).
Network admin, freelance web developer and editor at SitePoint .
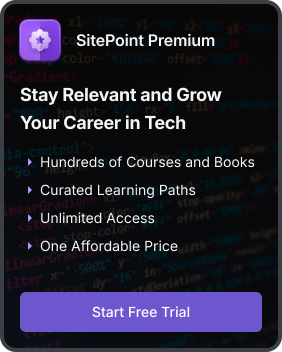
JavaScript Assignment Operators
JavaScript Assignment Operators | Example | Explanation |
---|---|---|
= | x = 15 | Value 15 is assigned to x |
+= | x += 15 | This is same as x = x + 15 |
-= | x -= 15 | This is same as x = x – 15 |
*= | x *= 15 | This is same as x = x * 15 |
/= | x /= 15 | This is same as x = x / 15 |
%= | x %= 15 | This is same as x = x % 15 |
JavaScript Assignment Operators Example
Javascript assignment operators example 2.
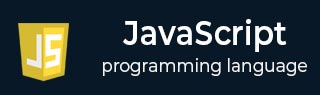
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Garbage Collection
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- ECMAScript 2023
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Generators
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Random Number
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - Export and Import
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Execution Context
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Design Patterns
- JavaScript - Reference Type
- JavaScript - LocalStorage
- JavaScript - SessionStorage
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Common Mistakes
- JavaScript - Performance
- JavaScript - Best Practices
- JavaScript - Style Guide
- JavaScript - Ninja Code
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Resources
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
JavaScript Assignment Operators
The assignment operators in JavaScript are used to assign values to the variables. These are binary operators. An assignment operator takes two operands , assigns a value to the left operand based on the value of right operand. The left operand is always a variable and the right operand may be literal, variable or expression.
An assignment operator first evaluates the expression and then assign the value to the variable (left operand).
A simple assignment operator is equal (=) operator. In the JavaScript statement "let x = 10;", the = operator assigns 10 to the variable x.
We can combine a simple assignment operator with other type of operators such as arithmetic, logical, etc. to get compound assignment operators. Some arithmetic assignment operators are +=, -=, *=, /=, etc. The += operator performs addition operation on the operands and assign the result to the left hand operand.
Arithmetic Assignment Operators
In this section, we will cover simple assignment and arithmetic assignment operators. An arithmetic assignment operator performs arithmetic operation and assign the result to a variable. Following is the list of operators with example −
Assignment Operator | Example | Equivalent To |
---|---|---|
= (Assignment) | a = b | a = b |
+= (Addition Assignment) | a += b | a = a + b |
-= (Subtraction Assignment) | a -= b | a = a – b |
*= (Multiplication Assignment) | a *= b | a = a * b |
/= (Division Assignment) | a /= b | a = a / b |
%= (Remainder Assignment) | a %= b | a = a % b |
**= (Exponentiation Assignment) | a **= b | a = a ** b |
Simple Assignment (=) Operator
Below is an example of assignment chaining −
Addition Assignment (+=) Operator
The JavaScript addition assignment operator performs addition on the two operands and assigns the result to the left operand. Here addition may be numeric addition or string concatenation.
In the above statement, it adds values of b and x and assigns the result to x.
Example: Numeric Addition Assignment
Example: string concatenation assignment, subtraction assignment (-=) operator.
The subtraction assignment operator in JavaScript subtracts the value of right operand from the left operand and assigns the result to left operand (variable).
In the above statement, it subtracts b from x and assigns the result to x.
Multiplication Assignment (*=) Operator
The multiplication assignment operator in JavaScript multiplies the both operands and assign the result to the left operand.
In the above statement, it multiplies x and b and assigns the result to x.
Division Assignment (/=) Operator
This operator divides left operand by the right operand and assigns the result to left operand.
In the above statement, it divides x by b and assigns the result (quotient) to x.
Remainder Assignment (%=) Operator
The JavaScript remainder assignment operator performs the remainder operation on the operands and assigns the result to left operand.
In the above statement, it divides x by b and assigns the result (remainder) to x.
Exponentiation Assignment (**=) Operator
This operator performs exponentiation operation on the operands and assigns the result to left operand.
In the above statement, it computes x**b and assigns the result to x.
JavaScript Bitwise Assignment operators
A bitwise assignment operator performs bitwise operation on the operands and assign the result to a variable. These operations perform two operations, first a bitwise operation and second the simple assignment operation. Bitwise operation is done on bit-level. A bitwise operator treats both operands as 32-bit signed integers and perform the operation on corresponding bits of the operands. The simple assignment operator assigns result is to the variable (left operand).
Following is the list of operators with example −
Assignment Operator | Example | Equivalent To |
---|---|---|
&= (Bitwise AND Assignment) | a &= b | a = a & b |
|= (Bitwise OR Assignment) | a |= b | a = a | b |
^= (Bitwise XOR Assignment) | a ^= b | a = a ^ b |
Bitwise AND Assignment Operator
The JavaScript bitwise AND assignment (&=) operator performs bitwise AND operation on the operands and assigns the result to the left operand (variable).
In the above statement, it performs bitwise AND on x and b and assigns the result to the variable x.
Bitwise OR Assignment Operator
The JavaScript bitwise OR assignment (|=) operator performs bitwise OR operation on the operands and assigns the result to the left operand (variable).
In the above statement, it performs bitwise OR on x and b and assigns the result to the variable x.
Bitwise XOR Assignment Operator
The JavaScript bitwise XOR assignment (^=) operator performs bitwise XOR operation on the operands and assigns the result to the left operand (variable).
In the above statement, it performs bitwise XOR on x and b and assigns the result to the variable x.
JavaScript Shift Assignment Operators
A shift assignment operator performs bitwise shift operation on the operands and assign the result to a variable (left operand). These are a combinations two operators, the first bitwise shift operator and second the simple assignment operator.
Following is the list of the shift assignment operators with example −
Assignment Operator | Example | Equivalent To |
---|---|---|
<<= (Left Shift Assignment) | a <<= b | a = a << b |
>>= (Right Shift Assignment) | a >>= b | a = a >> b |
>>>= (Unsigned Right Shift Assignment) | a >>>= b | a = a >>> b |
Left Shift Assignment Operator
The JavaScript left shift assignment (<<=) operator performs left shift operation on the operands and assigns the result to the left operand (variable).
In the above statement, it performs left shift on x and b and assigns the result to the variable x.
Right Shift Assignment Operator
The JavaScript right shift assignment (>>=) operator performs right shift operation on the operands and assigns the result to the left operand (variable).
In the above statement, it performs right shift on x and b and assigns the result to the variable x.
Unsigned Right Shift Assignment Operator
The JavaScript unsigned right shift assignment (>>>=) operator performs unsigned right shift operation on the operands and assigns the result to the left operand (variable).
In the above statement, it performs unsigned right shift on x and b and assigns the result to the variable x.
JavaScript Logical Assignment operators
In JavaScript, a logical assignment operator performs a logical operation on the operands and assign the result to a variable (left operand). Each logical assignment operator is a combinations two operators, the first logical operator and second the simple assignment operator.
Following is the list of the logical assignment operators with example −
Assignment Operator | Example | Equivalent To |
---|---|---|
&&= (Logical AND Assignment) | a &&= b | a = a && b |
||= (Logical OR Assignment) | a ||= b | a = a || b |
??= (Nullish Coalescing Assignment) | a ??= b | a = a ?? b |
Home » JavaScript Tutorial » The Ultimate Guide to JavaScript Symbol
The Ultimate Guide to JavaScript Symbol
Summary : in this tutorial, you will learn about the JavaScript symbol primitive type and how to use the symbol effectively.
Creating symbols
ES6 added Symbol as a new primitive type. Unlike other primitive types such as number , boolean , null , undefined , and string , the symbol type doesn’t have a literal form.
To create a new symbol, you use the global Symbol() function as shown in this example:
The Symbol() function creates a new unique value each time you call it:
The Symbol() function accepts a description as an optional argument. The description argument will make your symbol more descriptive.
The following example creates two symbols: firstName and lastName .
You can access the symbol’s description property using the toString() method. The console.log() method calls the toString() method of the symbol implicitly as shown in the following example:
Since symbols are primitive values, you can use the typeof operator to check whether a variable is a symbol. ES6 extended typeof to return the symbol string when you pass in a symbol variable:
Since a symbol is a primitive value, if you attempt to create a symbol using the new operator, you will get an error:
Sharing symbols
ES6 provides you with a global symbol registry that allows you to share symbols globally. If you want to create a symbol that will be shared, you use the Symbol.for() method instead of calling the Symbol() function.
The Symbol.for() method accepts a single parameter that can be used for symbol’s description, as shown in the following example:
The Symbol.for() method first searches for the symbol with the ssn key in the global symbol registry. It returns the existing symbol if there is one. Otherwise, the Symbol.for() method creates a new symbol, registers it to the global symbol registry with the specified key, and returns the symbol.
Later, if you call the Symbol.for() method using the same key, the Symbol.for() method will return the existing symbol.
In this example, we used the Symbol.for() method to look up the symbol with the ssn key. Since the global symbol registry already contained it, the Symbol.for() method returned the existing symbol.
To get the key associated with a symbol, you use the Symbol.keyFor() method as shown in the following example:
If a symbol does not exist in the global symbol registry, the System.keyFor() method returns undefined .
Symbol usages
A) using symbols as unique values.
Whenever you use a string or a number in your code, you should use symbols instead. For example, you have to manage the status in the task management application.
Before ES6, you would use strings such as open , in progress , completed , canceled , and on hold to represent different statuses of a task. In ES6, you can use symbols as follows:
B) Using a symbol as the computed property name of an object
You can use symbols as computed property names. See the following example:
To get all the enumerable properties of an object, you use the Object.keys() method.
To get all properties of an object whether the properties are enumerable or not, you use the Object.getOwnPropertyNames() method.
To get all property symbols of an object, you use the Object.getOwnPropertySymbols() method, which has been added in ES6.
The Object.getOwnPropertySymbols() method returns an array of own property symbols from an object.
Well-known symbols
ES6 provides predefined symbols which are called well-known symbols. The well-known symbols represent the common behaviors in JavaScript. Each well-known symbol is a static property of the Symbol object.
Symbol.hasInstance
The Symbol.hasInstance is a symbol that changes the behavior of the instanceof operator. Typically, when you use the instanceof operator:
JavaScript will call the Symbol.hasIntance method as follows:
It then depends on the method to determine if obj is an instance of the type object. See the following example.
The [] array is not an instance of the Stack class, therefore, the instanceof operator returns false in this example.
Assuming that you want the [] array is an instance of the Stack class, you can add the Symbol.hasInstance method as follows:
Symbol.iterator
The Symbol.iterator specifies whether a function will return an iterator for an object.
The objects that have Symbol.iterator property are called iterable objects.
In ES6, all collection objects (A rray , Set and Map ) and strings are iterable objects.
ES6 provides the for…of loop that works with the iterable object as in the following example.
Internally, the JavaScript engine first calls the Symbol.iterator method of the numbers array to get the iterator object.
Then, it invokes the iterator.next() method and copies the value property of the iterator object into the num variable.
After three iterations, the done property of the result object is true , the loop exits.
You can access the default iterator object via System.iterator symbol as follows:
By default, a collection is not iterable. However, you can make it iterable by using the Symbol.iterator as shown in the following example:
Symbol.isConcatSpreadable
To concatenate two arrays, you use the concat() method as shown in the following example:
In this example, the resulting array contains the single elements of both arrays. In addition, the concat() method also accepts a non-array argument as illustrated below.
The number 5 becomes the fifth element of the array.
As you can see in the above example when we pass an array to the concat() method, the concat() method spreads the array into individual elements. However, it treats a single primitive argument differently. Prior to ES6, you could not change this behavior.
This is why the Symbol.isConcatSpreadable symbol comes into play.
The Symbol.isConcatSpreadable property is a Boolean value that determines whether an object is added individually to the result of the concat() function.
Consider the following example:
The list object is concatenated to the ['Learning'] array. However, its individual elements are not spreaded.
To enable the elements of the list object added to the array individually when passing to the concat() method, you need to add the Symbol.isConcatSpreadable property to the list object as follows:
Note that if you set the value of the Symbol.isConcatSpreadable to false and pass the list object to the concat() method, it will be concatenated to the array as the whole object.
Symbol.toPrimitive
The Symbol.toPrimitive method determines what should happen when an object is converted into a primitive value.
The JavaScript engine defines the Symbol.toPrimitive method on the prototype of each standard type.
The Symbol.toPrimitive method takes a hint argument that has one of three values: “number”, “string”, and “default”. The hint argument specifies the type of the return value. The hint parameter is filled by the JavaScript engine based on the context in which the object is used.
Here is an example of using the Symbol.toPrimitive method.
In this tutorial, you have learned about JavaScript symbols and how to use symbols for unique values and object properties. Also, you learned how to use well-known symbols to modify object behaviors.

A Professional Coder‘s Deep Dive on JavaScript Symbols

As a full-stack developer with over 10 years of JavaScript experience, symbols have become an essential part of my toolkit. Introduced in ES6, JavaScript symbols provide a way to generate unique identifiers and prevent accidental name collisions – a common pitfall in large-scale development.
In this comprehensive 3200+ word guide, we‘ll cover:
- The problem symbols were designed to solve
- How symbols work under the hood to ensure uniqueness
- Various use cases including preventing name collisions, private members, iterators, and more
- Industry statistics on symbol adoption and usage
- Downsides and tradeoffs compared to alternatives like namespaces
- When symbols may (and may not) be appropriate for your codebase
I‘ll draw upon real-world examples from complex enterprise applications to demonstrate symbols in action across JavaScript‘s ecosystem. Let‘s dive in!
The Genesis of JavaScript Symbols
First, why were symbols added to JavaScript and what specific problem do they address?
The JavaScript ecosystem has continued to grow dramatically . Since its standardization with ES5 in 2009, adoption has skyrocketed to 97% of all websites today . Alongside this growth has come increasing code complexity and team scaling challenges .
With more developers working across shared codebases, accidental naming collisions became a nagging issue :
This problem intensified as modern JavaScript trends like module bundling, functional programming, and microservice architectures took hold. Even meticulous naming conventions broke down at scale.
Symbols arrived in ES6 as an abstraction to guarantee uniqueness for identifiers, constants, and private object members. Much like UUIDs in databases, symbols remove the burden of manually coordinating uniqueness. Instead, the engine handles it automatically under the hood.
But how exactly do symbols deliver on this promise of uniqueness while remaining performant?
Symbols Under the Hood
When invoking Symbol() , the spec mandates creation of a new and unique symbol on each call:
Implementations handle this requirement by automatically generating a fresh numeric identifier each time:
This incremental id assignment continues perpetually, ensuring symbols remain distinct without coordination.
Interestingly, V8 and SpiderMonkey took slightly different implementation routes :
V8 seeds each numeric id with a random hash prefix for added entropy and uniqueness in memory.
SpiderMonkey seeds numeric ids randomly without hashing.
But in both engines, automatic fresh value generation delivers on the spec‘s contract.
These implementation details matter because they allow symbols to provide uniqueness guarantees without compromising runtime performance . Automatic id generation avoids an expensive coordination lookup on each call.
Now equipped with an understanding of how symbols deliver uniqueness, let‘s explore various use cases taking advantage of this capability.
Use Case 1: Avoiding Name Collisions in Large Codebases
Without symbols, carefully coordinating unique names across large engineering orgs becomes untenable. Identifiers frequently clash:
While naming conventions help, they breakdown at scale and across teams. Interfaces also provide isolation but require refactors and can hamper reuse.
Symbols neatly sidestep name collisions without refactoring existing code:
This avoids coordination overhead while preserving readability.
Beyond a certain codebase complexity threshold, symbols deliver tangible maintainability and velocity improvements from avoided collisions. Most scale-phase companies like Facebook and Airbnb have adopted them as a result.
Use Case 2: Private Object Members
Symbols also commonly denote private object members in JavaScript:
This guarantees external code cannot accidentally access private fields, without needing explicit language support:
Note that symbols don‘t provide true privacy (nothing does in JS). But they effectively communicate intent of privacy.
This technique sees widespread adoption and will become even more common as private class fields ( # ) broaden usage.
Use Case 3: Extending Built-In Prototypes
Although riskier if handled incorrectly, symbols even allow extending native prototypes like:
Here a new forEachAsync method is added using a symbol key. Because the symbol key is unique, there‘s no risk of accidentally overriding an existing method.
This works reliably but should be handled with care:
- Prefer composition over extension when possible
- Carefully review conflicts with future proposals
- Rigorously test across environments
When applied judiciously, though, symbols facilitate useful extensions to native prototypes.
Use Case 4: Unique Constants and Enums
Symbols can also effectively create constants with unique identities :
The auto-uniqueness removes traditional constant coordination problems:
- Accidentally duplicating values
- Overwriting existing names
- Requiring a central constants registry
This centralized constants use case demonstrates further symbol adoption.
Industry Adoption and Usage
In fact, recent surveys substantiate growing industry adoption of symbols:

State of JS Data on Symbol Usage
Over 50% of respondents now use symbols in production code, with 83% recognizing them. This aligns with internal metrics I‘ve seen at Fortune 500 companies as well.
Beyond name collisions, iterators are the most common application (used heavily within React and Vue for example). But usage continues expanding across domains.
So in summary, while symbols carry a learning curve, they deliver tangible value in complex codebases. Understand and leverage them appropriately based on your scale and team dynamics.
Comparison: Tradeoffs vs Alternatives
Given increased complexity, are symbols always the best approach though? In some cases, alternatives like namespaces may suffice without the abstraction overhead.
Let‘s compare tradeoffs across approaches:

Symbols introduce more cognitive overhead for developers through new syntax, tooling gaps, and debuggability challenges. However, they also deliver the most reliable name isolation in complex codebases.
Alternatives like namespaces continue to play an important role where collision likelihood remains low. Evaluate complexity, extensibility needs, and team dynamics when deciding between approaches.
Conclusion: Apply Symbols Judiciously
JavaScript symbols serve as a uniquely valuable abstraction in the right circumstances. They provide failsafe name isolation and extendability guarantees unmatched by alternatives. However, these benefits come at the cost of added complexity.
Here are my guidelines on prudent symbol adoption:
- Default to strings for most identifiers until collisions become frequent
- Monitor collision incidents and refactoring overhead
- Transition to symbols when complexity crosses a project-specific threshold
- Ensure conventions and tooling support appropriate debugging
- Evaluate alternative approaches periodically as language capabilities evolve
I hope this deep dive has offered a seasoned full-stack developer‘s perspective on the why, how, and when of symbols. They‘ve become indispensable in my largest enterprise projects. Apply symbols judiciously based on your scale, team dynamics, and complexity trends.
Please reach out with any other symbol questions!
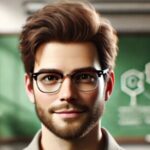
Dr. Alex Mitchell is a dedicated coding instructor with a deep passion for teaching and a wealth of experience in computer science education. As a university professor, Dr. Mitchell has played a pivotal role in shaping the coding skills of countless students, helping them navigate the intricate world of programming languages and software development.
Beyond the classroom, Dr. Mitchell is an active contributor to the freeCodeCamp community, where he regularly shares his expertise through tutorials, code examples, and practical insights. His teaching repertoire includes a wide range of languages and frameworks, such as Python, JavaScript, Next.js, and React, which he presents in an accessible and engaging manner.
Dr. Mitchell’s approach to teaching blends academic rigor with real-world applications, ensuring that his students not only understand the theory but also how to apply it effectively. His commitment to education and his ability to simplify complex topics have made him a respected figure in both the university and online learning communities.
Similar Posts

Getting Started with Kubernetes for your SaaS Application
Kubernetes has become the de facto standard for container orchestration, providing a robust platform to deploy,…

How to Configure Metadata for Single-Page Applications: An Expert Guide
Optimizing metadata is an essential task that can make or break the success of modern single-page…

How to set up Flutter Platform Channels with Protobuf
This post is an intermediate-advanced level guide aimed at audiences who want to write custom platform-specific…

Accessibility Best Practices – What to Remember When Building Accessible Web Apps
Building accessible web applications is crucial to ensure that people with disabilities can actively participate on…

Docker Cache – How to Do a Clean Image Rebuild and Clear Docker‘s Cache
Containers enable you to package your application in a portable way that can run in many…

A Cautionary Tale of Learning to Code. My own.
I was just a guy in a suit in an office with a vague startup idea….
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
- JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
- JavaScript Object.getOwnPropertySymbols()
JavaScript Iterators and Iterables
JavaScript typeof Operator
- JavaScript Object.getOwnPropertyDescriptor()
- JavaScript Object.hasOwnProperty()
JavaScript Data Types
The JavaScript ES6 introduced a new primitive data type called Symbol . Symbols are immutable (cannot be changed) and are unique. For example,
Though value1 and value2 both contain the same description, they are different.
- Creating Symbol
You use the Symbol() function to create a Symbol . For example,
You can pass an optional string as its description. For example,
- Access Symbol Description
To access the description of a symbol, we use the . operator. For example,
- Add Symbol as an Object Key
You can add symbols as a key in an object using square brackets [] . For example,
Symbols are not included in for...in Loop
The for...in loop does not iterate over Symbolic properties. For example,
- Benefit of Using Symbols in Object
If the same code snippet is used in various programs, then it is better to use Symbols in the object key. It's because you can use the same key name in different codes and avoid duplication issues. For example,
In the above program, if the person object is also used by another program, then you wouldn't want to add a property that can be accessed or changed by another program. Hence by using Symbol , you create a unique property that you can use.
Now, if the other program also needs to use a property named id , just add a Symbol named id and there won't be duplication issues. For example,
In the above program, even if the same name is used to store values, the Symbol data type will have a unique value.
In the above program, if the string key was used, then the later program would have changed the value of the property. For example,
In the above program, the second user.id overwrites the previous value.
- Symbol Methods
There are various methods available with Symbol.
Method | Description |
---|---|
Searches for existing symbols | |
Returns a shared symbol key from the global symbol registry. | |
Returns a string containing the source of the Symbol object | |
Returns a string containing the description of the Symbol | |
Returns the primitive value of the Symbol object. |
Example: Symbol Methods
- Symbol Properties
Properties | Description |
---|---|
Returns the default AsyncIterator for an object | |
Determines if a constructor object recognizes an object as its instance | |
Indicates if an object should be flattened to its array elements | |
Returns the default iterator for an object | |
Matches against a string | |
Returns an iterator that yields matches of the regular expression against a string | |
Replaces matched substrings of a string | |
Returns the index within a string that matches the regular expression | |
Splits a string at the indices that match a regular expression | |
Creates derived objects | |
Converts an object to a primitive value | |
Gives the default description of an object | |
Returns a string containing the description of the symbol |
Example: Symbol Properties Example
Table of contents.
- Symbols not included in for...in Loop
Sorry about that.
Related Tutorials
JavaScript Tutorial
JavaScript Regex
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
What does the construct x = x || y mean?
I am debugging some JavaScript and can't explain what this || does:
Why is this guy using var title = title || 'ERROR' ? I sometimes see it without a var declaration as well.
- optional-parameters
- or-operator

- 55 People have already answered this... but be extremely aware of the fact that the second value is chosen if the first value is falsy , not JUST undefined . The amount of times I've seen doWeDoIt = doWeDoIt || true , is enough to make me cry. (i.e doWeDoIt will now never be false ) – Matt Commented May 10, 2010 at 11:14
- 6 For those with experience with C#, the double-pipe operator is equivalent to null-coalesce operator ?? . Javascript evaluates non-null objects like true (or better evalualtes null objects to false) – usr-local-ΕΨΗΕΛΩΝ Commented Aug 27, 2015 at 13:32
- 4 Don't feel bad - JS is the only goofy language allowing this horrible coding....that and teaching that it's proper to nest every function into lines of code and throwing them away making them disposable and unusable a 2nd time. :) I'm 30 yrs into coding and held off touching JS until not long ago myself and I feel your pain all I can say is, keep a "makes no sense, it's only in JS" cheetsheet handy it's the only way I've gotten by! :) – Collin Chaffin Commented Apr 21, 2017 at 18:21
- 2 Please consider changing the accepted answer to my answer . – Michał Perłakowski Commented Mar 5, 2018 at 9:57
- 1 @usr-local-ΕΨΗΕΛΩΝ - I saw that date, and I covered that time frame: before that was added, the correct equivalent to null-coelesce was value != null ? value : YOUR_DEFAULT . Using || as if it was null coalesce was never a good habit. Subtle bug if you encountered any "falsey" value, but were thinking of it as "not null" rather than "not falsey". Maybe call || the "truthy coalesce operator"? :) – ToolmakerSteve Commented Oct 27, 2020 at 16:53
12 Answers 12
What is the double pipe operator ( || ).
The double pipe operator ( || ) is the logical OR operator . In most languages it works the following way:
- If the first value is false , it checks the second value. If that's true , it returns true and if the second value is false , it returns false .
- If the first value is true , it always returns true , no matter what the second value is.
So basically it works like this function:
If you still don't understand, look at this table:
In other words, it's only false when both values are false.
How is it different in JavaScript?
JavaScript is a bit different, because it's a loosely typed language . In this case it means that you can use || operator with values that are not booleans. Though it makes no sense, you can use this operator with for example a function and an object:
What happens there?
If values are not boolean, JavaScript makes implicit conversion to boolean . It means that if the value is falsey (e.g. 0 , "" , null , undefined (see also All falsey values in JavaScript )), it will be treated as false ; otherwise it's treated as true .
So the above example should give true , because empty function is truthy. Well, it doesn't. It returns the empty function. That's because JavaScript's || operator doesn't work as I wrote at the beginning. It works the following way:
- If the first value is falsey , it returns the second value .
- If the first value is truthy , it returns the first value .
Surprised? Actually, it's "compatible" with the traditional || operator. It could be written as following function:
If you pass a truthy value as x , it returns x , that is, a truthy value. So if you use it later in if clause:
you get "Either x or y is truthy." .
If x was falsey, eitherXorY would be y . In this case you would get the "Either x or y is truthy." if y was truthy; otherwise you'd get "Neither x nor y is truthy" .
The actual question
Now, when you know how || operator works, you can probably make out by yourself what does x = x || y mean. If x is truthy, x is assigned to x , so actually nothing happens; otherwise y is assigned to x . It is commonly used to define default parameters in functions. However, it is often considered a bad programming practice , because it prevents you from passing a falsey value (which is not necessarily undefined or null ) as a parameter. Consider following example:
It looks valid at the first sight. However, what would happen if you passed false as flagA parameter (since it's boolean, i.e. can be true or false )? It would become true . In this example, there is no way to set flagA to false .
It would be a better idea to explicitly check whether flagA is undefined , like that:
Though it's longer, it always works and it's easier to understand.
You can also use the ES6 syntax for default function parameters , but note that it doesn't work in older browsers (like IE). If you want to support these browsers, you should transpile your code with Babel .
See also Logical Operators on MDN .

- 21 +1 - by far the most correct and complete answer. And, for those with this question (some of us veteran coders new to JS included) should certainly focus the most out of this entire answer on this line: "Though it makes no sense" because this "loosley typed" will simply never make sense to those of us that grew up without it. For us, a boolean operator is just that and ONLY that......and whomever ever thought it would be a good idea to have to stop and think through some wacky conversion of non-boolean values to boolean while reading/writing code, forgot to take their meds that day! :) – Collin Chaffin Commented Apr 21, 2017 at 18:33
- 3 +1, in a nutshell: title = title || 'Error' means if (title) { title = title; } else { title = 'Error'; } – Andre Elrico Commented Feb 19, 2019 at 16:58
- I actually don't agree with the "though it makes no sense" line. I understand the line would not compile in C, for example, but it is well-understood if you came from Ruby for example, or even Groovy. In Ruby you could express it even shorter, as title ||= 'Error' . – Elliot Nelson Commented Dec 17, 2019 at 5:18
- FWIW, I would have written the code snippet as function or(x, y) { if (x) return x; else return y; } . This is essentially what is done in all languages AFAIK, both strict and loosely typed, including JavaScript. [ignoring short-circuiting for this discussion] The only difference between different languages is under what conditions if (x) is true. [In a strictly typed language, if (x) is only true for true , so return x is identical to return true .] – ToolmakerSteve Commented Oct 27, 2020 at 16:35
- 1 Revisited in 2021: use the nullish coalescing operator ( ?? ) – user4945014 Commented May 15, 2021 at 1:41
It means the title argument is optional. So if you call the method with no arguments it will use a default value of "Error" .
It's shorthand for writing:
This kind of shorthand trick with boolean expressions is common in Perl too. With the expression:
it evaluates to true if either a or b is true . So if a is true you don't need to check b at all. This is called short-circuit boolean evaluation so:
basically checks if title evaluates to false . If it does, it "returns" "Error" , otherwise it returns title .
- 5 Sorry to be picky, but the argument isn't optional, the argument is checked – themightybun Commented Oct 25, 2016 at 17:30
- 5 This is NOT the answer and I agree with the last comment it's not even optional. No part of this answer is correct even the Perl reference since the Perl statement actually makes SENSE and is evaluated in a completely different way. The JS is eval in a much more "converted" boolean logic method that I too find much more confusing to read/write. The answer below titled "What is the double pipe operator" is actually a correct answer. – Collin Chaffin Commented Apr 21, 2017 at 18:27
If title is not set, use 'ERROR' as default value.
More generic:
Reads: Set foobar to foo or default . You could even chain this up many times:
- 1 I found it confusing because the variables have the same name. Much easier when they don't. – Norbert Norbertson Commented Jun 29, 2017 at 12:47
Explaining this a little more...
The || operator is the logical- or operator. The result is true if the first part is true and it is true if the second part is true and it is true if both parts are true. For clarity, here it is in a table:
Now notice something here? If X is true, the result is always true. So if we know that X is true we don't have to check Y at all. Many languages thus implement "short circuit" evaluators for logical- or (and logical- and coming from the other direction). They check the first element and if that's true they don't bother checking the second at all. The result (in logical terms) is the same, but in terms of execution there's potentially a huge difference if the second element is expensive to calculate.
So what does this have to do with your example?
Let's look at that. The title element is passed in to your function. In JavaScript if you don't pass in a parameter, it defaults to a null value. Also in JavaScript if your variable is a null value it is considered to be false by the logical operators. So if this function is called with a title given, it is a non-false value and thus assigned to the local variable. If, however, it is not given a value, it is a null value and thus false. The logical- or operator then evaluates the second expression and returns 'Error' instead. So now the local variable is given the value 'Error'.
This works because of the implementation of logical expressions in JavaScript. It doesn't return a proper boolean value ( true or false ) but instead returns the value it was given under some rules as to what's considered equivalent to true and what's considered equivalent to false . Look up your JavaScript reference to learn what JavaScript considers to be true or false in boolean contexts.
|| is the boolean OR operator. As in JavaScript, undefined, null, 0, false are considered as falsy values.
It simply means

Basically, it checks if the value before the || evaluates to true. If yes, it takes this value, and if not, it takes the value after the || .
Values for which it will take the value after the || (as far as I remember):
- '' (Null or Null string)
- 1 false || null || undefined || 0 || '' || 'you forgot null' – Dziamid Commented May 16, 2013 at 15:02
Whilst Cletus' answer is correct, I feel more detail should be added in regards to "evaluates to false" in JavaScript.
Is not just checking if title/msg has been provided, but also if either of them are falsy . i.e. one of the following:
false. 0 (zero) "" (empty string) null. undefined. NaN (a special Number value meaning Not-a-Number!)
So in the line
If title is truthy (i.e., not falsy, so title = "titleMessage" etc.) then the Boolean OR (||) operator has found one 'true' value, which means it evaluates to true, so it short-circuits and returns the true value (title).
If title is falsy (i.e. one of the list above), then the Boolean OR (||) operator has found a 'false' value, and now needs to evaluate the other part of the operator, 'Error', which evaluates to true, and is hence returned.
It would also seem (after some quick firebug console experimentation) if both sides of the operator evaluate to false, it returns the second 'falsy' operator.
returns undefined, this is probably to allow you to use the behavior asked about in this question when trying to default title/message to "". i.e. after running
foo would be set to ""
Double pipe stands for logical "OR". This is not really the case when the "parameter not set", since strictly in JavaScript if you have code like this:
are not equivalent.
Double pipe (||) will cast the first argument to Boolean and if the resulting Boolean is true - do the assignment, otherwise it will assign the right part.
This matters if you check for unset parameter.
Let's say, we have a function setSalary that has one optional parameter. If the user does not supply the parameter then the default value of 10 should be used.
If you do the check like this:
This will give an unexpected result for a call like:
It will still set the 10 following the flow described above.
Double pipe operator
This example may be useful:
It can also be:
To add some explanation to all said before me, I should give you some examples to understand logical concepts.
It means if the left side evaluated as a true statement it will be finished and the left side will be returned and assigned to the variable. in other cases the right side will be returned and assigned.
And operator have the opposite structure like below.
Quote: "What does the construct x = x || y mean?"
Assigning a default value.
This means providing a default value of y to x , in case x is still waiting for its value but hasn't received it yet or was deliberately omitted in order to fall back to a default.

- That's the exact meaning of the construct and the only meaning of it. And it was vastly as a subroutine in writing functions that could be fetched as prototypes, standalone functions, and also as borrowed methods to be applied on another element. Where its main and only duty was to modify the reference of the target. Example: function getKeys(x) { x = x || this ; .... } which could be used without modification as a standalone function, as a property method in prototypes and as a method of an element which can get another element as its argument as ` [element].getKeys(anotherElement);` – Bekim Bacaj Commented May 18, 2016 at 2:34
And I have to add one more thing: This bit of shorthand is an abomination. It misuses an accidental interpreter optimization (not bothering with the second operation if the first is truthy) to control an assignment. That use has nothing to do with the purpose of the operator. I do not believe it should ever be used.
I prefer the ternary operator for initialization, for example,
This uses a one-line conditional operation for its correct purpose. It still plays unsightly games with truthiness but, that's JavaScript for you.

- Short-circuit Boolean operators were criticised by Edsger W. Dijkstra and others, but they go a long time back and are in a lot of languages , including C, Pascal (Turbo Pascal only?), Perl, C#, Go , Kotlin , Python, etc. For instance, Python only has short-circuit Boolean operators. – Peter Mortensen Commented May 1, 2021 at 2:36
Not the answer you're looking for? Browse other questions tagged javascript parameters optional-parameters or-operator or ask your own question .
- The Overflow Blog
- Ryan Dahl explains why Deno had to evolve with version 2.0
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
- What does a new user need in a homepage experience on Stack Overflow?
Hot Network Questions
- Which BASIC dialect first featured a single-character comment introducer?
- Find all pairs of positive compatible numbers less than 100 by proof
- On intersection theory on toric varieties
- How can an Investigator get critical specialization effects on unarmed attacks?
- I can't select a certain record with like %value%
- Is there a word/phrase that describes things people say to be "the smartest person in the room"?
- My first actual cryptic
- Does "any computer(s) you have" refer to one or all the computers?
- The beloved shepherd isn't Solomon?
- Why would an incumbent politician or party need to be re-elected to fulfill a campaign promise?
- Inconsistent width of relational operators in newtxmath
- What might cause these striations in this solder joint?
- What is the rationale behind requiring ATC to retire at age 56?
- Has any spacecraft ever been severely damaged by a micrometeorite?
- The Master Tetrist
- Why name the staves in LilyPond's "published" "Solo piano" template?
- Can I remove duplicate capacitors while merging two modules?
- Seven different digits are placed in a row. The products of the first 3, middle 3 and last 3 are all equal. What is the middle digit?
- Arranging people in a jeep.
- result of a union between a decidable language and not recognizable one - disjoint
- How soon to fire rude and chaotic PhD student?
- The meaning of “manage” in this context
- How many people could we get off of the planet in a month?
- Home water pressure higher than city water pressure?
Skip header and go to main content
Open Data Portal beta
Open Data Portal will be moving
A new Open Data Portal (ODP) is launching soon! Developer Hub will continue to run in parallel with the new ODP through 2025. Thank you for your help in moving us out of beta. Learn more about the new Open Data Portal on data.uspto.gov .
USPTO Datasets
Protecting inventors and entrepreneurs fuels innovation and creativity, driving advances that can benefit society. as the federal agency that grants patents and registers trademarks, we hold a treasure trove of data. now we’re giving it to you - faster and easier than before., the artificial intelligence patent dataset (stata (.dta) and ms excel (.csv)), patent assignment economics data for academia and researchers: created/maintained by the uspto chief economist (jan 1970 - dec 2022).
- Patent assignment economics data (stata (.dta) and MS excel…
- Cancer Moonshot data (MS excel (.csv))
- Patent application Office actions data (stata (.dta) and MS…
- Patent Litigation data (stata (.dta) and MS Excel (.csv))
- Patent examination research dataset (stata (.dta) and MS…
- Patent and patent application Claims data (Stata (.dta) and…
- The Artificial Intelligence Patent Dataset (Stata (.dta)…
Latest Release
Documents & resources.
The zip files here also contain resources.

IMAGES
COMMENTS
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
Use the correct assignment operator that will result in x being 15 (same as x = x + y ). Start the Exercise. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that ...
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. ... JavaScript Assignment Operators. Assignment operators assign values to JavaScript variables. The Addition Assignment Operator (+=) adds a value to a variable.
An assignment operator ( =) assigns a value to a variable. The syntax of the assignment operator is as follows: let a = b; Code language: JavaScript (javascript) In this syntax, JavaScript evaluates the expression b first and assigns the result to the variable a. The following example declares the counter variable and initializes its value to zero:
JavaScript Exponentiation Assignment Operator in JavaScript is represented by "**=". This operator is used to raise the value of the variable to the power of the operand which is right. This can also be explained as the first variable is the power of the second operand. The exponentiation operator is equal to Math.pow(). Syntax: a **= b or a = a **
JavaScript Operators. Operators are used to assign values, compare values, perform arithmetic operations, and more. There are different types of JavaScript operators: Arithmetic Operators. Assignment Operators. Comparison Operators. Logical Operators. Conditional Operators. Type Operators.
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript. Assignment Operators. In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
JavaScript operators are special symbols that perform operations on one or more operands (values). In this tutorial, you will learn about JavaScript operators with the help of examples. ... JavaScript Assignment Operators. We use assignment operators to assign values to variables. For example, let x = 5; Here, we used the = operator to assign ...
The assignment operator in JavaScript is used to assign values to variables. It is represented by the "=" symbol. For example, if you have a variable named "x" and you want to assign the ...
The JavaScript Assignment operators are used to assign values to the declared variables. Equals (=) operator is the most commonly used assignment operator. For example: var i = 10; The below table displays all the JavaScript assignment operators. JavaScript Assignment Operators. Example. Explanation. =.
A simple assignment operator is equal (=) operator. In the JavaScript statement "let x = 10;", the = operator assigns 10 to the variable x. We can combine a simple assignment operator with other type of operators such as arithmetic, logical, etc. to get compound assignment operators. Some arithmetic assignment operators are +=, -=, *=, /=, etc.
Summary: in this tutorial, you will learn about the JavaScript symbol primitive type and how to use the symbol effectively.. Creating symbols. ES6 added Symbol as a new primitive type. Unlike other primitive types such as number, boolean, null, undefined, and string, the symbol type doesn't have a literal form.. To create a new symbol, you use the global Symbol() function as shown in this ...
Symbol() // Symbol(1) Symbol() // Symbol(2) Symbol('mySymbol') // Symbol(3) This incremental id assignment continues perpetually, ensuring symbols remain distinct without coordination. Interestingly, V8 ... JavaScript symbols serve as a uniquely valuable abstraction in the right circumstances. They provide failsafe name isolation and ...
Basic keywords and general expressions in JavaScript. These expressions have the highest precedence (higher than operators ). The this keyword refers to a special property of an execution context. Basic null, boolean, number, and string literals. Array initializer/literal syntax. Object initializer/literal syntax.
Symbol is a built-in object whose constructor returns a symbol primitive — also called a Symbol value or just a Symbol — that's guaranteed to be unique. Symbols are often used to add unique property keys to an object that won't collide with keys any other code might add to the object, and which are hidden from any mechanisms other code will typically use to access the object. That enables ...
It's a little hard to google when all you have are symbols ;) The terms to use are "JavaScript conditional operator". If you see any more funny symbols in JavaScript, you should try looking up JavaScript's operators first: Mozilla Developer Center's list of operators. The one exception you're likely to encounter is the $ symbol.
The JavaScript ES6 introduced a new primitive data type called Symbol. Symbols are immutable (cannot be changed) and are unique. For example, // two symbols with the same description const value1 = Symbol('hello'); const value2 = Symbol('hello'); console.log(value1 === value2); // false. Though value1 and value2 both contain the same ...
In JavaScript if you don't pass in a parameter, it defaults to a null value. Also in JavaScript if your variable is a null value it is considered to be false by the logical operators. So if this function is called with a title given, it is a non-false value and thus assigned to the local variable.
The logical OR assignment ( ||=) operator only evaluates the right operand and assigns to the left if the left operand is falsy. Try it. Syntax. js. x ||= y. Description. Logical OR assignment short-circuits, meaning that x ||= y is equivalent to x || (x = y), except that the expression x is only evaluated once.
Patent assignment economics data for academia and researchers: created/maintained by the USPTO Chief Economist (JAN 1970 - DEC 2022) Back to Results. Contains detailed information on roughly 10.0 million patent assignments and other transactions recorded at the USPTO since 1970 and involving roughly 17.8 million patents and patent applications ...
Logical AND assignment (&&=) The logical AND assignment ( &&=) operator only evaluates the right operand and assigns to the left if the left operand is truthy. Try it. Syntax. js. x &&= y. Description. Logical AND assignment short-circuits, meaning that x &&= y is equivalent to x && (x = y), except that the expression x is only evaluated once.