Browse Course Material
Course info.
- Sarina Canelake

Departments
- Electrical Engineering and Computer Science
As Taught In
- Programming Languages
- Software Design and Engineering
Learning Resource Types
A gentle introduction to programming using python, assignments.
If you are working on your own machine, you will probably need to install Python. We will be using the standard Python software, available here . You should download and install version 2.6.x, not 2.7.x or 3.x. All MIT Course 6 classes currently use a version of Python 2.6.
ASSN # | ASSIGNMENTS | SUPPORTING FILES |
---|---|---|
Homework 1 | Handout ( ) Written exercises ( ) | Code template ( ) |
Homework 2 | Handout ( ) Written exercises ( ) | Code template ( ) nims.py ( ) strings_and_lists.py ( ) |
Project 1: Hangman | Handout ( ) | hangman_template.py ( ) words.txt ( ) Optional extension: hangman_lib.py ( ) hangman_lib_demo.py ( ) |
Homework 3 | Handout ( ) Written exercises ( ) | Code template ( ) |
Homework 4 | Handout ( ) Written exercises ( ) | Graphics module documentation ( ) graphics.py ( ) — be sure to save this in the same directory where your code is saved! wheel.py ( ) rgb.txt ( ) The graphics.py package and documentation are courtesy of John Zelle, and are used with permission. |
Project 2: Conway’s game of life | Handout ( ) | game_of_life_template.py ( ) — download and save as game_of_life.py; be sure to save in the same directory as graphics.py |
Final project: Tetris | Handout ( ) | tetris_template.py ( ) — Download and save it in the same directory as graphics.py. Please edit this file, and place your code in the sections that say “YOUR CODE HERE”. |

You are leaving MIT OpenCourseWare
35 Python Programming Exercises and Solutions
To understand a programming language deeply, you need to practice what you’ve learned. If you’ve completed learning the syntax of Python programming language, it is the right time to do some practice programs.
1. Python program to check whether the given number is even or not.
2. python program to convert the temperature in degree centigrade to fahrenheit, 3. python program to find the area of a triangle whose sides are given, 4. python program to find out the average of a set of integers, 5. python program to find the product of a set of real numbers, 6. python program to find the circumference and area of a circle with a given radius, 7. python program to check whether the given integer is a multiple of 5, 8. python program to check whether the given integer is a multiple of both 5 and 7, 9. python program to find the average of 10 numbers using while loop, 10. python program to display the given integer in a reverse manner, 11. python program to find the geometric mean of n numbers, 12. python program to find the sum of the digits of an integer using a while loop, 13. python program to display all the multiples of 3 within the range 10 to 50, 14. python program to display all integers within the range 100-200 whose sum of digits is an even number, 15. python program to check whether the given integer is a prime number or not, 16. python program to generate the prime numbers from 1 to n, 17. python program to find the roots of a quadratic equation, 18. python program to print the numbers from a given number n till 0 using recursion, 19. python program to find the factorial of a number using recursion, 20. python program to display the sum of n numbers using a list, 21. python program to implement linear search, 22. python program to implement binary search, 23. python program to find the odd numbers in an array, 24. python program to find the largest number in a list without using built-in functions, 25. python program to insert a number to any position in a list, 26. python program to delete an element from a list by index, 27. python program to check whether a string is palindrome or not, 28. python program to implement matrix addition, 29. python program to implement matrix multiplication, 30. python program to check leap year, 31. python program to find the nth term in a fibonacci series using recursion, 32. python program to print fibonacci series using iteration, 33. python program to print all the items in a dictionary, 34. python program to implement a calculator to do basic operations, 35. python program to draw a circle of squares using turtle.
For practicing more such exercises, I suggest you go to hackerrank.com and sign up. You’ll be able to practice Python there very effectively.
I hope these exercises were helpful to you. If you have any doubts, feel free to let me know in the comments.
12 thoughts on “ 35 Python Programming Exercises and Solutions ”
I don’t mean to nitpick and I don’t want this published but you might want to check code for #16. 4 is not a prime number.
You can only check if integer is a multiple of 35. It always works the same – just multiply all the numbers you need to check for multiplicity.
v_str = str ( input(‘ Enter a valid string or number :- ‘) ) v_rev_str=” for v_d in v_str: v_rev_str = v_d + v_rev_str
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Recent Posts
Python Programming
Python Basic Exercise for Beginners
Updated on: August 31, 2023 | 497 Comments
This Python essential exercise is to help Python beginners to learn necessary Python skills quickly.
Immerse yourself in the practice of Python’s foundational concepts, such as loops, control flow, data types, operators, list, strings, input-output, and built-in functions. This beginner’s exercise is certain to elevate your understanding of Python.
Also, See :
- Python Quizzes : Solve quizzes to check your knowledge of fundamental concepts.
- Python Basics : Learn the basics to solve this exercise.
What questions are included in this Python fundamental exercise ?
- The exercise contains 15 programs to solve. The hint and solution is provided for each question.
- I have added tips and required learning resources for each question, which will help you solve the exercise. When you complete each question, you get more familiar with the basics of Python.
Use Online Code Editor to solve exercise questions.
This Python exercise covers questions on the following topics :
- Python for loop and while loop
- Python list , set , tuple , dictionary , input, and output
Also, try to solve the basic Python Quiz for beginners
Exercise 1: Calculate the multiplication and sum of two numbers
Given two integer numbers, return their product only if the product is equal to or lower than 1000. Otherwise, return their sum.
Expected Output :
- Accept user input in Python
- Calculate an Average in Python
- Create a function that will take two numbers as parameters
- Next, Inside a function, multiply two numbers and save their product in a product variable
- Next, use the if condition to check if the product >1000 . If yes, return the product
- Otherwise, use the else block to calculate the sum of two numbers and return it.
Exercise 2: Print the sum of the current number and the previous number
Write a program to iterate the first 10 numbers, and in each iteration, print the sum of the current and previous number.
Reference article for help:
- Python range() function
- Calculate sum and average in Python
- Create a variable called previous_num and assign it to 0
- Iterate the first 10 numbers one by one using for loop and range() function
- Next, display the current number ( i ), previous number, and the addition of both numbers in each iteration of the loop. Finally, change the value of the previous number to the current number ( previous_num = i ).
Exercise 3: Print characters from a string that are present at an even index number
Write a program to accept a string from the user and display characters that are present at an even index number.
For example, str = "pynative" so you should display ‘p’, ‘n’, ‘t’, ‘v’.
Reference article for help: Python Input and Output
- Use the Python input() function to accept a string from a user.
- Calculate the length of the string using the len() function
- Next, iterate each character of a string using for loop and range() function.
- Use start = 0 , stop = len(s)-1, and step =2 . the step is 2 because we want only even index numbers
- In each iteration of a loop, use s[i] to print characters present at the current even index number
Solution 1 :
Solution 2 : Using list slicing
Exercise 4: Remove first n characters from a string
Write a program to remove characters from a string starting from zero up to n and return a new string.
For example:
- remove_chars("pynative", 4) so output must be tive . Here, we need to remove the first four characters from a string.
- remove_chars("pynative", 2) so output must be native . Here, we need to remove the first two characters from a string.
Note : n must be less than the length of the string.
Use string slicing to get the substring. For example, to remove the first four characters and the remaining use s[4:] .
Also, try to solve Python String Exercise
Exercise 5: Check if the first and last number of a list is the same
Write a function to return True if the first and last number of a given list is same. If numbers are different then return False .
Exercise 6: Display numbers divisible by 5 from a list
Iterate the given list of numbers and print only those numbers which are divisible by 5
Also, try to solve Python list Exercise
Exercise 7: Return the count of a given substring from a string
Write a program to find how many times substring “ Emma ” appears in the given string.
Use string method count() .
Solution 1 : Use the count() method
Solution 2 : Without string method
Exercise 8: Print the following pattern
Hint : Print Pattern using for loop
Exercise 9: Check Palindrome Number
Write a program to check if the given number is a palindrome number.
A palindrome number is a number that is the same after reverse. For example, 545, is the palindrome numbers
- Reverse the given number and save it in a different variable
- Use the if condition to check if the original and reverse numbers are identical. If yes, return True .
Exercise 10: Create a new list from two list using the following condition
Create a new list from two list using the following condition
Given two list of numbers, write a program to create a new list such that the new list should contain odd numbers from the first list and even numbers from the second list.
- Create an empty list named result_list
- Iterate the first list using a for loop
- In each iteration, check if the current number is odd number using num % 2 != 0 formula. If the current number is an odd number, add it to the result list
- Now, Iterate the first list using a loop.
- In each iteration, check if the current number is odd number using num % 2 == 0 formula. If the current number is an even number, add it to the result list
- Print the result list
Note: Try to solve the Python list Exercise
Exercise 11: Write a Program to extract each digit from an integer in the reverse order.
For example, If the given int is 7536 , the output shall be “ 6 3 5 7 “, with a space separating the digits.
Use while loop
Exercise 12: Calculate income tax for the given income by adhering to the rules below
Taxable Income | Rate (in %) |
---|---|
First $10,000 | 0 |
Next $10,000 | 10 |
The remaining | 20 |
For example, suppose the taxable income is 45000, and the income tax payable is
10000*0% + 10000*10% + 25000*20% = $6000.
Exercise 13: Print multiplication table from 1 to 10
See: How two use nested loops in Python
- Create the outer for loop to iterate numbers from 1 to 10. So, the total number of iterations of the outer loop is 10.
- Create an inner loop to iterate 10 times.
- For each outer loop iteration, the inner loop will execute ten times.
- In the first iteration of the nested loop, the number is 1. In the next, it 2. and so on till 10.
- In each iteration of an inner loop, we calculated the multiplication of two numbers. (current outer number and current inner number)
Exercise 14: Print a downward Half-Pyramid Pattern of Star (asterisk)
Exercise 15: write a function called exponent(base, exp) that returns an int value of base raises to the power of exp..
Note here exp is a non-negative integer, and the base is an integer.
Expected output
I want to hear from you. What do you think of this basic exercise? If you have better alternative answers to the above questions, please help others by commenting on this exercise.
I have shown only 15 questions in this exercise because we have Topic-specific exercises to cover each topic exercise in detail. Please solve all Python exercises .
Did you find this page helpful? Let others know about it. Sharing helps me continue to create free Python resources.
About Vishal

I’m Vishal Hule , the Founder of PYnative.com. As a Python developer, I enjoy assisting students, developers, and learners. Follow me on Twitter .
Related Tutorial Topics:
Python exercises and quizzes.
Free coding exercises and quizzes cover Python basics, data structure, data analytics, and more.
- 15+ Topic-specific Exercises and Quizzes
- Each Exercise contains 10 questions
- Each Quiz contains 12-15 MCQ
Loading comments... Please wait.
About PYnative
PYnative.com is for Python lovers. Here, You can get Tutorials, Exercises, and Quizzes to practice and improve your Python skills .
Explore Python
- Learn Python
- Python Basics
- Python Databases
- Python Exercises
- Python Quizzes
- Online Python Code Editor
- Python Tricks
To get New Python Tutorials, Exercises, and Quizzes
Legal Stuff
We use cookies to improve your experience. While using PYnative, you agree to have read and accepted our Terms Of Use , Cookie Policy , and Privacy Policy .
Copyright © 2018–2024 pynative.com
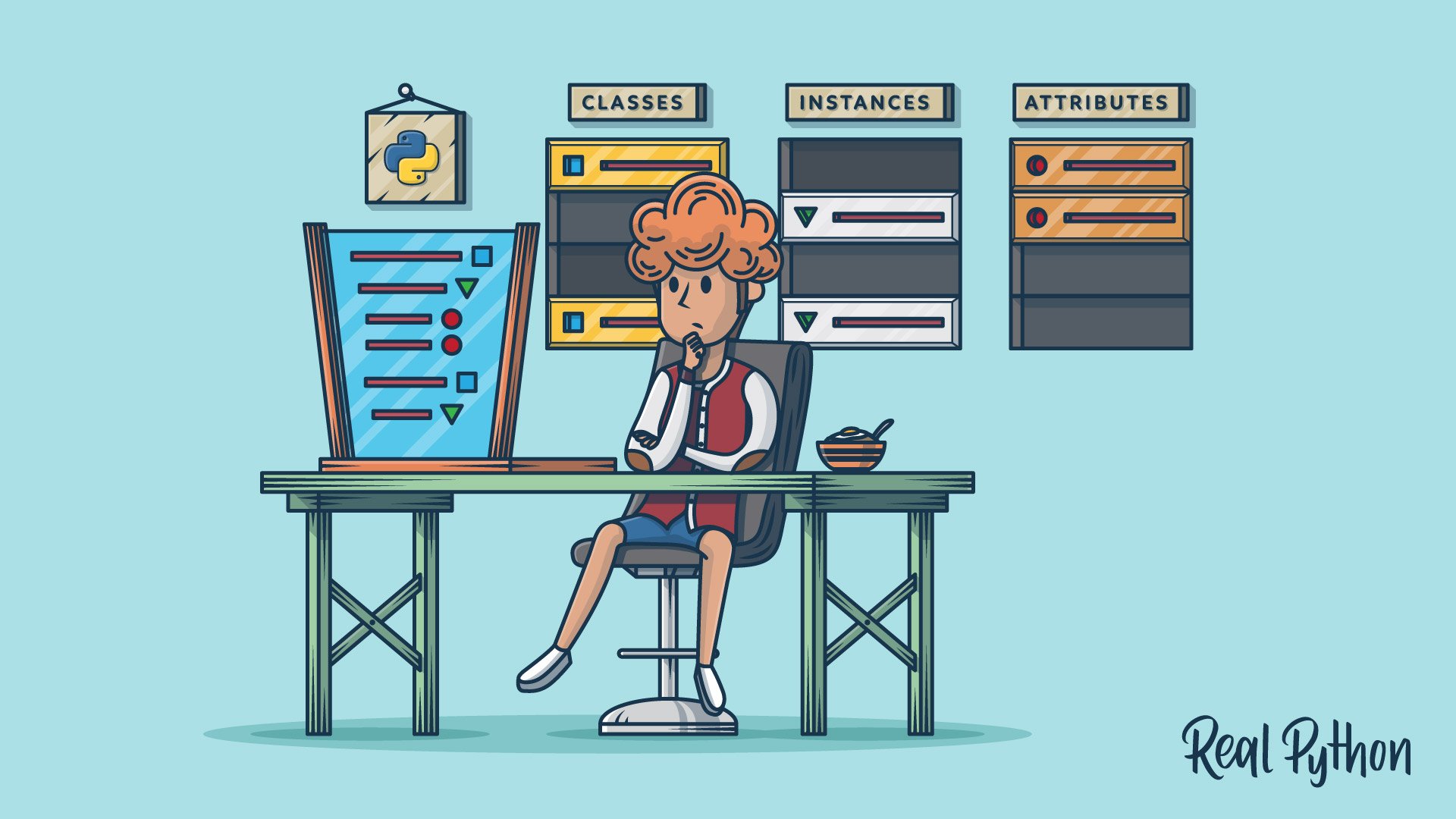
Object-Oriented Programming (OOP) in Python
Table of Contents
What Is Object-Oriented Programming in Python?
Classes vs instances, class definition, class and instance attributes, instance methods, example: dog park, parent classes vs child classes, parent class functionality extension.
Watch Now This tutorial has a related video course created by the Real Python team. Watch it together with the written tutorial to deepen your understanding: Intro to Object-Oriented Programming (OOP) in Python
Object-oriented programming (OOP) is a method of structuring a program by bundling related properties and behaviors into individual objects . In this tutorial, you’ll learn the basics of object-oriented programming in Python.
Conceptually, objects are like the components of a system. Think of a program as a factory assembly line of sorts. At each step of the assembly line, a system component processes some material, ultimately transforming raw material into a finished product.
An object contains data, like the raw or preprocessed materials at each step on an assembly line. In addition, the object contains behavior, like the action that each assembly line component performs.
In this tutorial, you’ll learn how to:
- Define a class , which is like a blueprint for creating an object
- Use classes to create new objects
- Model systems with class inheritance
Note: This tutorial is adapted from the chapter “Object-Oriented Programming (OOP)” in Python Basics: A Practical Introduction to Python 3 .
The book uses Python’s built-in IDLE editor to create and edit Python files and interact with the Python shell, so you’ll see occasional references to IDLE throughout this tutorial. If you don’t use IDLE, you can run the example code from the editor and environment of your choice.
Get Your Code: Click here to download the free sample code that shows you how to do object-oriented programming with classes in Python 3.
Take the Quiz: Test your knowledge with our interactive “Object-Oriented Programming (OOP) in Python 3” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Object-oriented programming (OOP) is a method of structuring a program by bundling related properties and behaviors into individual objects.
Object-oriented programming is a programming paradigm that provides a means of structuring programs so that properties and behaviors are bundled into individual objects .
For example, an object could represent a person with properties like a name, age, and address and behaviors such as walking, talking, breathing, and running. Or it could represent an email with properties like a recipient list, subject, and body and behaviors like adding attachments and sending.
Put another way, object-oriented programming is an approach for modeling concrete, real-world things, like cars, as well as relations between things, like companies and employees or students and teachers. OOP models real-world entities as software objects that have some data associated with them and can perform certain operations.
Note: You can also check out the Python Basics: Object-Oriented Programming video course to reinforce the skills that you’ll develop in this section of the tutorial.
The key takeaway is that objects are at the center of object-oriented programming in Python. In other programming paradigms, objects only represent the data. In OOP, they additionally inform the overall structure of the program.
How Do You Define a Class in Python?
In Python, you define a class by using the class keyword followed by a name and a colon. Then you use .__init__() to declare which attributes each instance of the class should have:
But what does all of that mean? And why do you even need classes in the first place? Take a step back and consider using built-in, primitive data structures as an alternative.
Primitive data structures—like numbers , strings , and lists —are designed to represent straightforward pieces of information, such as the cost of an apple, the name of a poem, or your favorite colors, respectively. What if you want to represent something more complex?
For example, you might want to track employees in an organization. You need to store some basic information about each employee, such as their name, age, position, and the year they started working.
One way to do this is to represent each employee as a list :
There are a number of issues with this approach.
First, it can make larger code files more difficult to manage. If you reference kirk[0] several lines away from where you declared the kirk list, will you remember that the element with index 0 is the employee’s name?
Second, it can introduce errors if employees don’t have the same number of elements in their respective lists. In the mccoy list above, the age is missing, so mccoy[1] will return "Chief Medical Officer" instead of Dr. McCoy’s age.
A great way to make this type of code more manageable and more maintainable is to use classes .
Classes allow you to create user-defined data structures. Classes define functions called methods , which identify the behaviors and actions that an object created from the class can perform with its data.
In this tutorial, you’ll create a Dog class that stores some information about the characteristics and behaviors that an individual dog can have.
A class is a blueprint for how to define something. It doesn’t actually contain any data. The Dog class specifies that a name and an age are necessary for defining a dog, but it doesn’t contain the name or age of any specific dog.
While the class is the blueprint, an instance is an object that’s built from a class and contains real data. An instance of the Dog class is not a blueprint anymore. It’s an actual dog with a name, like Miles, who’s four years old.
Put another way, a class is like a form or questionnaire. An instance is like a form that you’ve filled out with information. Just like many people can fill out the same form with their own unique information, you can create many instances from a single class.
You start all class definitions with the class keyword, then add the name of the class and a colon. Python will consider any code that you indent below the class definition as part of the class’s body.
Here’s an example of a Dog class:
The body of the Dog class consists of a single statement: the pass keyword. Python programmers often use pass as a placeholder indicating where code will eventually go. It allows you to run this code without Python throwing an error.
Note: Python class names are written in CapitalizedWords notation by convention. For example, a class for a specific breed of dog, like the Jack Russell Terrier, would be written as JackRussellTerrier .
The Dog class isn’t very interesting right now, so you’ll spruce it up a bit by defining some properties that all Dog objects should have. There are several properties that you can choose from, including name, age, coat color, and breed. To keep the example small in scope, you’ll just use name and age.
You define the properties that all Dog objects must have in a method called .__init__() . Every time you create a new Dog object, .__init__() sets the initial state of the object by assigning the values of the object’s properties. That is, .__init__() initializes each new instance of the class.
You can give .__init__() any number of parameters, but the first parameter will always be a variable called self . When you create a new class instance, then Python automatically passes the instance to the self parameter in .__init__() so that Python can define the new attributes on the object.
Update the Dog class with an .__init__() method that creates .name and .age attributes:
Make sure that you indent the .__init__() method’s signature by four spaces, and the body of the method by eight spaces. This indentation is vitally important. It tells Python that the .__init__() method belongs to the Dog class.
In the body of .__init__() , there are two statements using the self variable:
- self.name = name creates an attribute called name and assigns the value of the name parameter to it.
- self.age = age creates an attribute called age and assigns the value of the age parameter to it.
Attributes created in .__init__() are called instance attributes . An instance attribute’s value is specific to a particular instance of the class. All Dog objects have a name and an age, but the values for the name and age attributes will vary depending on the Dog instance.
On the other hand, class attributes are attributes that have the same value for all class instances. You can define a class attribute by assigning a value to a variable name outside of .__init__() .
For example, the following Dog class has a class attribute called species with the value "Canis familiaris" :
You define class attributes directly beneath the first line of the class name and indent them by four spaces. You always need to assign them an initial value. When you create an instance of the class, then Python automatically creates and assigns class attributes to their initial values.
Use class attributes to define properties that should have the same value for every class instance. Use instance attributes for properties that vary from one instance to another.
Now that you have a Dog class, it’s time to create some dogs!
How Do You Instantiate a Class in Python?
Creating a new object from a class is called instantiating a class. You can create a new object by typing the name of the class, followed by opening and closing parentheses:
You first create a new Dog class with no attributes or methods, and then you instantiate the Dog class to create a Dog object.
In the output above, you can see that you now have a new Dog object at 0x106702d30 . This funny-looking string of letters and numbers is a memory address that indicates where Python stores the Dog object in your computer’s memory. Note that the address on your screen will be different.
Now instantiate the Dog class a second time to create another Dog object:
The new Dog instance is located at a different memory address. That’s because it’s an entirely new instance and is completely unique from the first Dog object that you created.
To see this another way, type the following:
In this code, you create two new Dog objects and assign them to the variables a and b . When you compare a and b using the == operator, the result is False . Even though a and b are both instances of the Dog class, they represent two distinct objects in memory.
Now create a new Dog class with a class attribute called .species and two instance attributes called .name and .age :
To instantiate this Dog class, you need to provide values for name and age . If you don’t, then Python raises a TypeError :
To pass arguments to the name and age parameters, put values into the parentheses after the class name:
This creates two new Dog instances—one for a four-year-old dog named Miles and one for a nine-year-old dog named Buddy.
The Dog class’s .__init__() method has three parameters, so why are you only passing two arguments to it in the example?
When you instantiate the Dog class, Python creates a new instance of Dog and passes it to the first parameter of .__init__() . This essentially removes the self parameter, so you only need to worry about the name and age parameters.
Note: Behind the scenes, Python both creates and initializes a new object when you use this syntax. If you want to dive deeper, then you can read the dedicated tutorial about the Python class constructor .
After you create the Dog instances, you can access their instance attributes using dot notation :
You can access class attributes the same way:
One of the biggest advantages of using classes to organize data is that instances are guaranteed to have the attributes you expect. All Dog instances have .species , .name , and .age attributes, so you can use those attributes with confidence, knowing that they’ll always return a value.
Although the attributes are guaranteed to exist, their values can change dynamically:
In this example, you change the .age attribute of the buddy object to 10 . Then you change the .species attribute of the miles object to "Felis silvestris" , which is a species of cat. That makes Miles a pretty strange dog, but it’s valid Python!
The key takeaway here is that custom objects are mutable by default. An object is mutable if you can alter it dynamically. For example, lists and dictionaries are mutable, but strings and tuples are immutable .
Instance methods are functions that you define inside a class and can only call on an instance of that class. Just like .__init__() , an instance method always takes self as its first parameter.
Open a new editor window in IDLE and type in the following Dog class:
This Dog class has two instance methods:
- .description() returns a string displaying the name and age of the dog.
- .speak() has one parameter called sound and returns a string containing the dog’s name and the sound that the dog makes.
Save the modified Dog class to a file called dog.py and press F5 to run the program. Then open the interactive window and type the following to see your instance methods in action:
In the above Dog class, .description() returns a string containing information about the Dog instance miles . When writing your own classes, it’s a good idea to have a method that returns a string containing useful information about an instance of the class. However, .description() isn’t the most Pythonic way of doing this.
When you create a list object, you can use print() to display a string that looks like the list:
Go ahead and print the miles object to see what output you get:
When you print miles , you get a cryptic-looking message telling you that miles is a Dog object at the memory address 0x00aeff70 . This message isn’t very helpful. You can change what gets printed by defining a special instance method called .__str__() .
In the editor window, change the name of the Dog class’s .description() method to .__str__() :
Save the file and press F5 . Now, when you print miles , you get a much friendlier output:
Methods like .__init__() and .__str__() are called dunder methods because they begin and end with double underscores . There are many dunder methods that you can use to customize classes in Python. Understanding dunder methods is an important part of mastering object-oriented programming in Python, but for your first exploration of the topic, you’ll stick with these two dunder methods.
Note: Check out When Should You Use .__repr__() vs .__str__() in Python? to learn more about .__str__() and its cousin .__repr__() .
If you want to reinforce your understanding with a practical exercise, then you can click on the block below and work on solving the challenge:
Exercise: Create a Car Class Show/Hide
Create a Car class with two instance attributes:
- .color , which stores the name of the car’s color as a string
- .mileage , which stores the number of miles on the car as an integer
Then create two Car objects—a blue car with twenty thousand miles and a red car with thirty thousand miles—and print out their colors and mileage. Your output should look like this:
There are multiple ways to solve this challenge. To effectively practice what you’ve learned so far, try to solve the task with the information about classes in Python that you’ve gathered in this section.
When you’re done with your own implementation of the challenge, then you can expand the block below to see a possible solution:
Solution: Create a Car Class Show/Hide
First, create a Car class with .color and .mileage instance attributes, and a .__str__() method to format the display of objects when you pass them to print() :
The color and mileage parameters of .__init__() are assigned to self.color and self.mileage , which creates the two instance attributes.
The .__str__() method interpolates both instance attributes into an f-string and uses the :, format specifier to print the mileage grouped by thousands and separated with a comma.
Now you can create the two Car instances:
You create the blue_car instance by passing the value "blue" to the color parameter and 20_000 to the mileage parameter. Similarly, you create red_car with the values "red" and 30_000 .
To print the color and mileage of each Car object, you can loop over a tuple containing both objects and print each object:
Because you’ve defined their string representation in .__str__() , printing the objects gives you the desired text output.
When you’re ready, you can move on to the next section. There, you’ll see how to take your knowledge one step further and create classes from other classes.
How Do You Inherit From Another Class in Python?
Inheritance is the process by which one class takes on the attributes and methods of another. Newly formed classes are called child classes , and the classes that you derive child classes from are called parent classes .
You inherit from a parent class by creating a new class and putting the name of the parent class into parentheses:
In this minimal example, the child class Child inherits from the parent class Parent . Because child classes take on the attributes and methods of parent classes, Child.hair_color is also "brown" without your explicitly defining that.
Note: This tutorial is adapted from the chapter “Object-Oriented Programming (OOP)” in Python Basics: A Practical Introduction to Python 3 . If you enjoy what you’re reading, then be sure to check out the rest of the book and the learning path .
You can also check out the Python Basics: Building Systems With Classes video course to reinforce the skills that you’ll develop in this section of the tutorial.
Child classes can override or extend the attributes and methods of parent classes. In other words, child classes inherit all of the parent’s attributes and methods but can also specify attributes and methods that are unique to themselves.
Although the analogy isn’t perfect, you can think of object inheritance sort of like genetic inheritance.
You may have inherited your hair color from your parents. It’s an attribute that you were born with. But maybe you decide to color your hair purple. Assuming that your parents don’t have purple hair, you’ve just overridden the hair color attribute that you inherited from your parents:
If you change the code example like this, then Child.hair_color will be "purple" .
You also inherit, in a sense, your language from your parents. If your parents speak English, then you’ll also speak English. Now imagine you decide to learn a second language, like German. In this case, you’ve extended your attributes because you’ve added an attribute that your parents don’t have:
You’ll learn more about how the code above works in the sections below. But before you dive deeper into inheritance in Python, you’ll take a walk to a dog park to better understand why you might want to use inheritance in your own code.
Pretend for a moment that you’re at a dog park. There are many dogs of different breeds at the park, all engaging in various dog behaviors.
Suppose now that you want to model the dog park with Python classes. The Dog class that you wrote in the previous section can distinguish dogs by name and age but not by breed.
You could modify the Dog class in the editor window by adding a .breed attribute:
Press F5 to save the file. Now you can model the dog park by creating a bunch of different dogs in the interactive window:
Each breed of dog has slightly different behaviors. For example, bulldogs have a low bark that sounds like woof , but dachshunds have a higher-pitched bark that sounds more like yap .
Using just the Dog class, you must supply a string for the sound argument of .speak() every time you call it on a Dog instance:
Passing a string to every call to .speak() is repetitive and inconvenient. Moreover, the .breed attribute should determine the string representing the sound that each Dog instance makes, but here you have to manually pass the correct string to .speak() every time you call it.
You can simplify the experience of working with the Dog class by creating a child class for each breed of dog. This allows you to extend the functionality that each child class inherits, including specifying a default argument for .speak() .
In this section, you’ll create a child class for each of the three breeds mentioned above: Jack Russell terrier, dachshund, and bulldog.
For reference, here’s the full definition of the Dog class that you’re currently working with:
After doing the dog park example in the previous section, you’ve removed .breed again. You’ll now write code to keep track of a dog’s breed using child classes instead.
To create a child class, you create a new class with its own name and then put the name of the parent class in parentheses. Add the following to the dog.py file to create three new child classes of the Dog class:
Press F5 to save and run the file. With the child classes defined, you can now create some dogs of specific breeds in the interactive window:
Instances of child classes inherit all of the attributes and methods of the parent class:
To determine which class a given object belongs to, you can use the built-in type() :
What if you want to determine if miles is also an instance of the Dog class? You can do this with the built-in isinstance() :
Notice that isinstance() takes two arguments, an object and a class. In the example above, isinstance() checks if miles is an instance of the Dog class and returns True .
The miles , buddy , jack , and jim objects are all Dog instances, but miles isn’t a Bulldog instance, and jack isn’t a Dachshund instance:
More generally, all objects created from a child class are instances of the parent class, although they may not be instances of other child classes.
Now that you’ve created child classes for some different breeds of dogs, you can give each breed its own sound.
Since different breeds of dogs have slightly different barks, you want to provide a default value for the sound argument of their respective .speak() methods. To do this, you need to override .speak() in the class definition for each breed.
To override a method defined on the parent class, you define a method with the same name on the child class. Here’s what that looks like for the JackRussellTerrier class:
Now .speak() is defined on the JackRussellTerrier class with the default argument for sound set to "Arf" .
Update dog.py with the new JackRussellTerrier class and press F5 to save and run the file. You can now call .speak() on a JackRussellTerrier instance without passing an argument to sound :
Sometimes dogs make different noises, so if Miles gets angry and growls, you can still call .speak() with a different sound:
One thing to keep in mind about class inheritance is that changes to the parent class automatically propagate to child classes. This occurs as long as the attribute or method being changed isn’t overridden in the child class.
For example, in the editor window, change the string returned by .speak() in the Dog class:
Save the file and press F5 . Now, when you create a new Bulldog instance named jim , jim.speak() returns the new string:
However, calling .speak() on a JackRussellTerrier instance won’t show the new style of output:
Sometimes it makes sense to completely override a method from a parent class. But in this case, you don’t want the JackRussellTerrier class to lose any changes that you might make to the formatting of the Dog.speak() output string.
To do this, you still need to define a .speak() method on the child JackRussellTerrier class. But instead of explicitly defining the output string, you need to call the Dog class’s .speak() from inside the child class’s .speak() using the same arguments that you passed to JackRussellTerrier.speak() .
You can access the parent class from inside a method of a child class by using super() :
When you call super().speak(sound) inside JackRussellTerrier , Python searches the parent class, Dog , for a .speak() method and calls it with the variable sound .
Update dog.py with the new JackRussellTerrier class. Save the file and press F5 so you can test it in the interactive window:
Now when you call miles.speak() , you’ll see output reflecting the new formatting in the Dog class.
Note: In the above examples, the class hierarchy is very straightforward. The JackRussellTerrier class has a single parent class, Dog . In real-world examples, the class hierarchy can get quite complicated.
The super() function does much more than just search the parent class for a method or an attribute. It traverses the entire class hierarchy for a matching method or attribute. If you aren’t careful, super() can have surprising results.
If you want to check your understanding of the concepts that you learned about in this section with a practical exercise, then you can click on the block below and work on solving the challenge:
Exercise: Class Inheritance Show/Hide
Start with the following code for your parent Dog class:
Create a GoldenRetriever class that inherits from the Dog class. Give the sound argument of GoldenRetriever.speak() a default value of "Bark" .
Solution: Class Inheritance Show/Hide
Create a class called GoldenRetriever that inherits from the Dog class and overrides the .speak() method:
You give "Bark" as the default value to the sound parameter in GoldenRetriever.speak() . Then you use super() to call the .speak() method of the parent class with the same argument passed to sound as the GoldenRetriever class’s .speak() method.
Nice work! In this section, you’ve learned how to override and extend methods from a parent class, and you worked on a small practical example to cement your new skills.
In this tutorial, you learned about object-oriented programming (OOP) in Python. Most modern programming languages, such as Java , C# , and C++ , follow OOP principles, so the knowledge that you gained here will be applicable no matter where your programming career takes you.
In this tutorial, you learned how to:
- Define a class , which is a sort of blueprint for an object
- Instantiate a class to create an object
- Use attributes and methods to define the properties and behaviors of an object
- Use inheritance to create child classes from a parent class
- Reference a method on a parent class using super()
- Check if an object inherits from another class using isinstance()
If you enjoyed what you learned in this sample from Python Basics: A Practical Introduction to Python 3 , then be sure to check out the rest of the book and check out our introduction to Python learning path.
🐍 Python Tricks 💌
Get a short & sweet Python Trick delivered to your inbox every couple of days. No spam ever. Unsubscribe any time. Curated by the Real Python team.
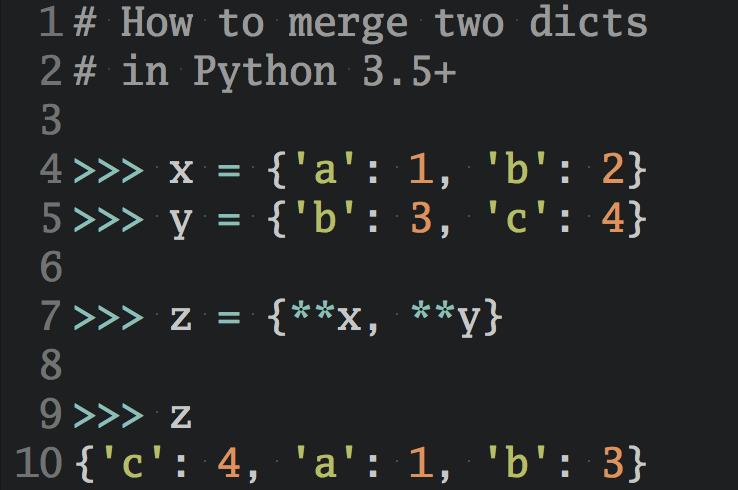
About David Amos
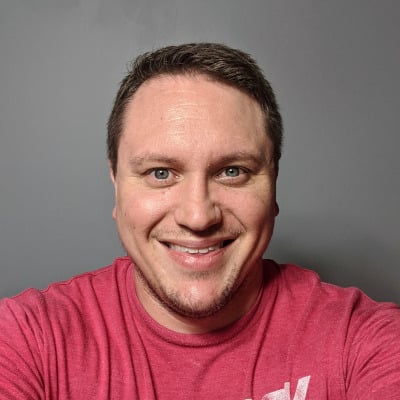
David is a writer, programmer, and mathematician passionate about exploring mathematics through code.
Each tutorial at Real Python is created by a team of developers so that it meets our high quality standards. The team members who worked on this tutorial are:
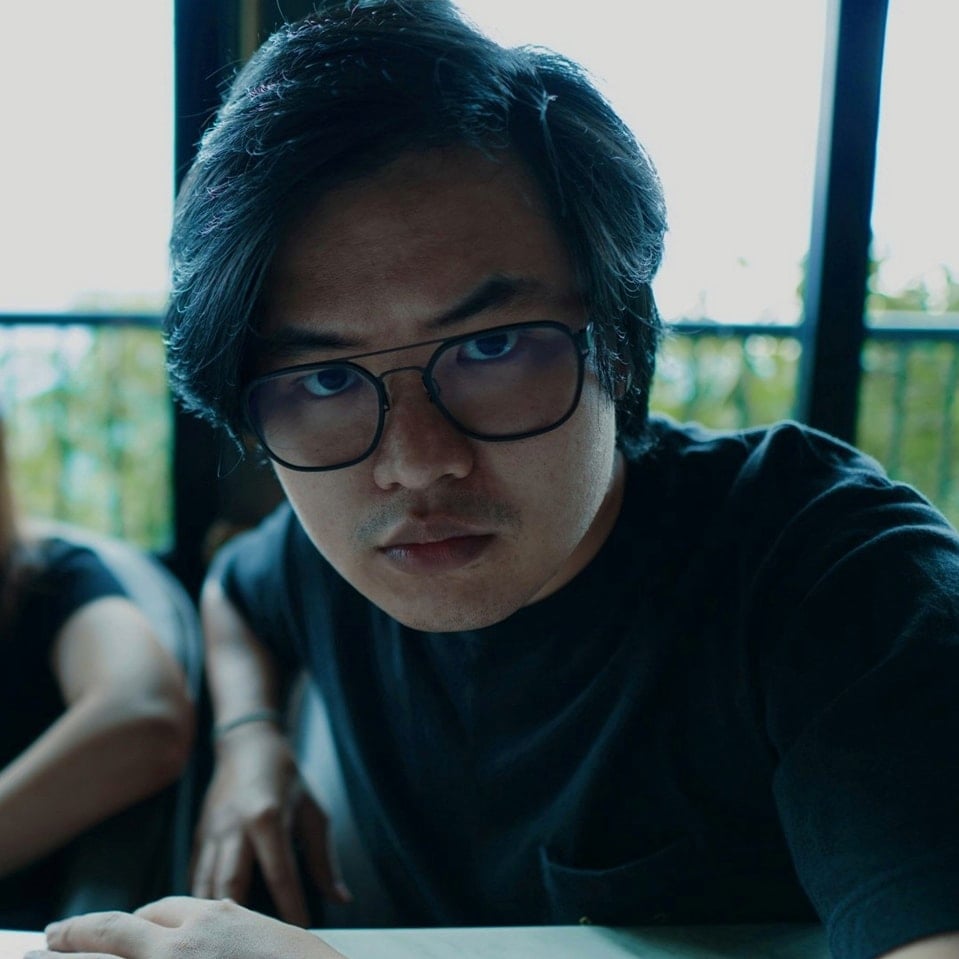
Master Real-World Python Skills With Unlimited Access to Real Python
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
What Do You Think?
What’s your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment below and let us know.
Commenting Tips: The most useful comments are those written with the goal of learning from or helping out other students. Get tips for asking good questions and get answers to common questions in our support portal . Looking for a real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session . Happy Pythoning!
Keep Learning
Related Topics: intermediate python
Recommended Video Course: Intro to Object-Oriented Programming (OOP) in Python
Keep reading Real Python by creating a free account or signing in:
Already have an account? Sign-In
Almost there! Complete this form and click the button below to gain instant access:
Object-Oriented Programming in Python 3 (Sample Code)
🔒 No spam. We take your privacy seriously.
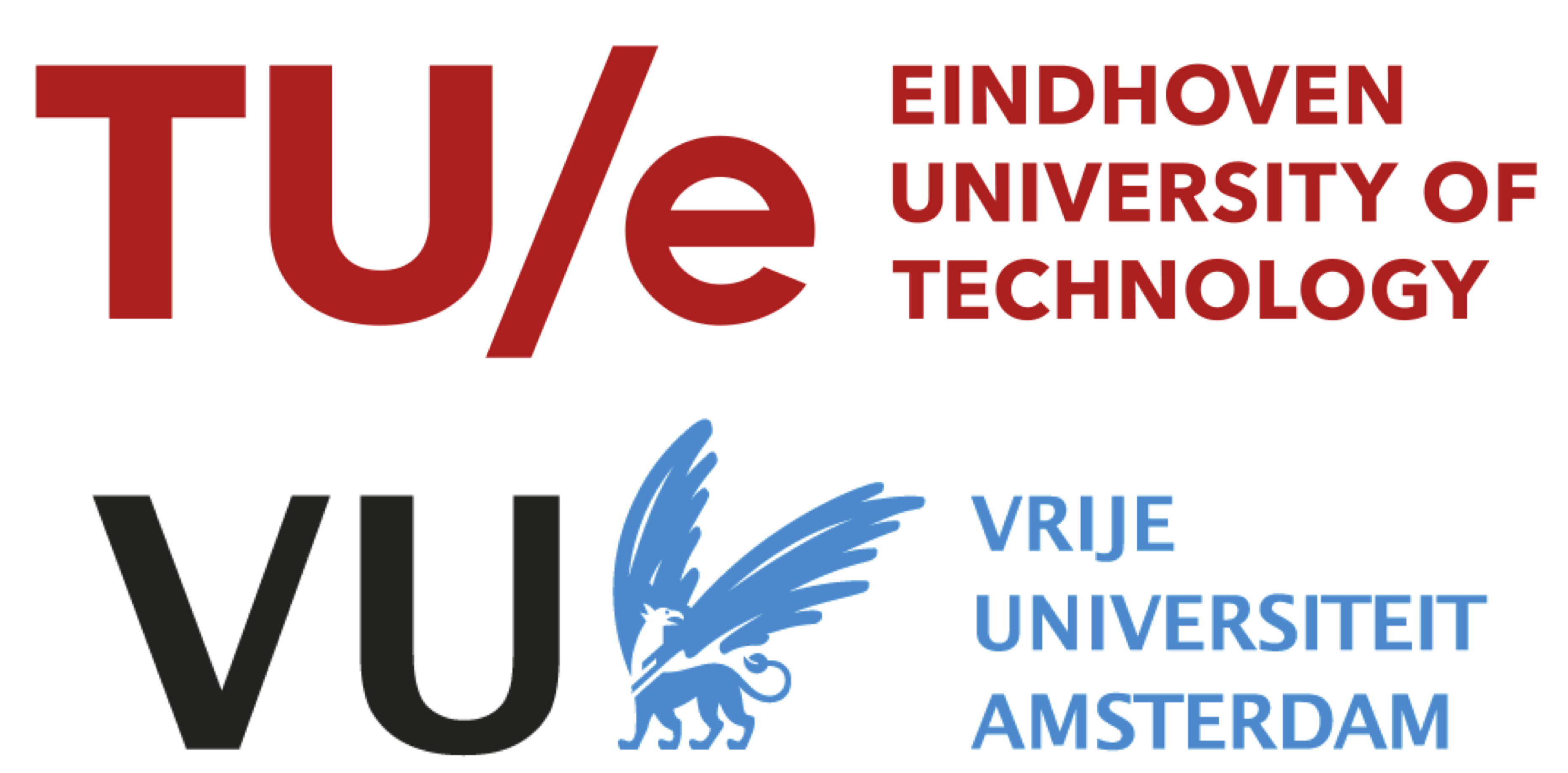
Learning Python by doing
- suggest edit
Variables, Expressions, and Assignments
Variables, expressions, and assignments 1 #, introduction #.
In this chapter, we introduce some of the main building blocks needed to create programs–that is, variables, expressions, and assignments. Programming related variables can be intepret in the same way that we interpret mathematical variables, as elements that store values that can later be changed. Usually, variables and values are used within the so-called expressions. Once again, just as in mathematics, an expression is a construct of values and variables connected with operators that result in a new value. Lastly, an assignment is a language construct know as an statement that assign a value (either as a constant or expression) to a variable. The rest of this notebook will dive into the main concepts that we need to fully understand these three language constructs.
Values and Types #
A value is the basic unit used in a program. It may be, for instance, a number respresenting temperature. It may be a string representing a word. Some values are 42, 42.0, and ‘Hello, Data Scientists!’.
Each value has its own type : 42 is an integer ( int in Python), 42.0 is a floating-point number ( float in Python), and ‘Hello, Data Scientists!’ is a string ( str in Python).
The Python interpreter can tell you the type of a value: the function type takes a value as argument and returns its corresponding type.
Observe the difference between type(42) and type('42') !
Expressions and Statements #
On the one hand, an expression is a combination of values, variables, and operators.
A value all by itself is considered an expression, and so is a variable.
When you type an expression at the prompt, the interpreter evaluates it, which means that it calculates the value of the expression and displays it.
In boxes above, m has the value 27 and m + 25 has the value 52 . m + 25 is said to be an expression.
On the other hand, a statement is an instruction that has an effect, like creating a variable or displaying a value.
The first statement initializes the variable n with the value 17 , this is a so-called assignment statement .
The second statement is a print statement that prints the value of the variable n .
The effect is not always visible. Assigning a value to a variable is not visible, but printing the value of a variable is.
Assignment Statements #
We have already seen that Python allows you to evaluate expressions, for instance 40 + 2 . It is very convenient if we are able to store the calculated value in some variable for future use. The latter can be done via an assignment statement. An assignment statement creates a new variable with a given name and assigns it a value.
The example in the previous code contains three assignments. The first one assigns the value of the expression 40 + 2 to a new variable called magicnumber ; the second one assigns the value of π to the variable pi , and; the last assignment assigns the string value 'Data is eatig the world' to the variable message .
Programmers generally choose names for their variables that are meaningful. In this way, they document what the variable is used for.
Do It Yourself!
Let’s compute the volume of a cube with side \(s = 5\) . Remember that the volume of a cube is defined as \(v = s^3\) . Assign the value to a variable called volume .
Well done! Now, why don’t you print the result in a message? It can say something like “The volume of the cube with side 5 is \(volume\) ”.
Beware that there is no checking of types ( type checking ) in Python, so a variable to which you have assigned an integer may be re-used as a float, even if we provide type-hints .
Names and Keywords #
Names of variable and other language constructs such as functions (we will cover this topic later), should be meaningful and reflect the purpose of the construct.
In general, Python names should adhere to the following rules:
It should start with a letter or underscore.
It cannot start with a number.
It must only contain alpha-numeric (i.e., letters a-z A-Z and digits 0-9) characters and underscores.
They cannot share the name of a Python keyword.
If you use illegal variable names you will get a syntax error.
By choosing the right variables names you make the code self-documenting, what is better the variable v or velocity ?
The following are examples of invalid variable names.
These basic development principles are sometimes called architectural rules . By defining and agreeing upon architectural rules you make it easier for you and your fellow developers to understand and modify your code.
If you want to read more on this, please have a look at Code complete a book by Steven McConnell [ McC04 ] .
Every programming language has a collection of reserved keywords . They are used in predefined language constructs, such as loops and conditionals . These language concepts and their usage will be explained later.
The interpreter uses keywords to recognize these language constructs in a program. Python 3 has the following keywords:
False class finally is return
None continue for lambda try
True def from nonlocal while
and del global not with
as elif if or yield
assert else import pass break
except in raise
Reassignments #
It is allowed to assign a new value to an existing variable. This process is called reassignment . As soon as you assign a value to a variable, the old value is lost.
The assignment of a variable to another variable, for instance b = a does not imply that if a is reassigned then b changes as well.
You have a variable salary that shows the weekly salary of an employee. However, you want to compute the monthly salary. Can you reassign the value to the salary variable according to the instruction?
Updating Variables #
A frequently used reassignment is for updating puposes: the value of a variable depends on the previous value of the variable.
This statement expresses “get the current value of x , add one, and then update x with the new value.”
Beware, that the variable should be initialized first, usually with a simple assignment.
Do you remember the salary excercise of the previous section (cf. 13. Reassignments)? Well, if you have not done it yet, update the salary variable by using its previous value.
Updating a variable by adding 1 is called an increment ; subtracting 1 is called a decrement . A shorthand way of doing is using += and -= , which stands for x = x + ... and x = x - ... respectively.
Order of Operations #
Expressions may contain multiple operators. The order of evaluation depends on the priorities of the operators also known as rules of precedence .
For mathematical operators, Python follows mathematical convention. The acronym PEMDAS is a useful way to remember the rules:
Parentheses have the highest precedence and can be used to force an expression to evaluate in the order you want. Since expressions in parentheses are evaluated first, 2 * (3 - 1) is 4 , and (1 + 1)**(5 - 2) is 8 . You can also use parentheses to make an expression easier to read, even if it does not change the result.
Exponentiation has the next highest precedence, so 1 + 2**3 is 9 , not 27 , and 2 * 3**2 is 18 , not 36 .
Multiplication and division have higher precedence than addition and subtraction . So 2 * 3 - 1 is 5 , not 4 , and 6 + 4 / 2 is 8 , not 5 .
Operators with the same precedence are evaluated from left to right (except exponentiation). So in the expression degrees / 2 * pi , the division happens first and the result is multiplied by pi . To divide by 2π, you can use parentheses or write: degrees / 2 / pi .
In case of doubt, use parentheses!
Let’s see what happens when we evaluate the following expressions. Just run the cell to check the resulting value.
Floor Division and Modulus Operators #
The floor division operator // divides two numbers and rounds down to an integer.
For example, suppose that driving to the south of France takes 555 minutes. You might want to know how long that is in hours.
Conventional division returns a floating-point number.
Hours are normally not represented with decimal points. Floor division returns the integer number of hours, dropping the fraction part.
You spend around 225 minutes every week on programming activities. You want to know around how many hours you invest to this activity during a month. Use the \(//\) operator to give the answer.
The modulus operator % works on integer values. It computes the remainder when dividing the first integer by the second one.
The modulus operator is more useful than it seems.
For example, you can check whether one number is divisible by another—if x % y is zero, then x is divisible by y .
String Operations #
In general, you cannot perform mathematical operations on strings, even if the strings look like numbers, so the following operations are illegal: '2'-'1' 'eggs'/'easy' 'third'*'a charm'
But there are two exceptions, + and * .
The + operator performs string concatenation, which means it joins the strings by linking them end-to-end.
The * operator also works on strings; it performs repetition.
Speedy Gonzales is a cartoon known to be the fastest mouse in all Mexico . He is also famous for saying “Arriba Arriba Andale Arriba Arriba Yepa”. Can you use the following variables, namely arriba , andale and yepa to print the mentioned expression? Don’t forget to use the string operators.
Asking the User for Input #
The programs we have written so far accept no input from the user.
To get data from the user through the Python prompt, we can use the built-in function input .
When input is called your whole program stops and waits for the user to enter the required data. Once the user types the value and presses Return or Enter , the function returns the input value as a string and the program continues with its execution.
Try it out!
You can also print a message to clarify the purpose of the required input as follows.
The resulting string can later be translated to a different type, like an integer or a float. To do so, you use the functions int and float , respectively. But be careful, the user might introduce a value that cannot be converted to the type you required.
We want to know the name of a user so we can display a welcome message in our program. The message should say something like “Hello \(name\) , welcome to our hello world program!”.
Script Mode #
So far we have run Python in interactive mode in these Jupyter notebooks, which means that you interact directly with the interpreter in the code cells . The interactive mode is a good way to get started, but if you are working with more than a few lines of code, it can be clumsy. The alternative is to save code in a file called a script and then run the interpreter in script mode to execute the script. By convention, Python scripts have names that end with .py .
Use the PyCharm icon in Anaconda Navigator to create and execute stand-alone Python scripts. Later in the course, you will have to work with Python projects for the assignments, in order to get acquainted with another way of interacing with Python code.
This Jupyter Notebook is based on Chapter 2 of the books Python for Everybody [ Sev16 ] and Think Python (Sections 5.1, 7.1, 7.2, and 5.12) [ Dow15 ] .
- Python Course
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Assignment Operators in Python
The Python Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, and bitwise computations. The value the operator operates on is known as the Operand. Here, we will cover Different Assignment operators in Python .
Operators |
| ||
---|---|---|---|
| = | Assign the value of the right side of the expression to the left side operand | c = a + b |
| += | Add right side operand with left side operand and then assign the result to left operand | a += b |
| -= | Subtract right side operand from left side operand and then assign the result to left operand | a -= b |
| *= | Multiply right operand with left operand and then assign the result to the left operand | a *= b |
| /= | Divide left operand with right operand and then assign the result to the left operand | a /= b |
| %= | Divides the left operand with the right operand and then assign the remainder to the left operand | a %= b |
| //= | Divide left operand with right operand and then assign the value(floor) to left operand | a //= b |
| **= | Calculate exponent(raise power) value using operands and then assign the result to left operand | a **= b |
| &= | Performs Bitwise AND on operands and assign the result to left operand | a &= b |
| |= | Performs Bitwise OR on operands and assign the value to left operand | a |= b |
| ^= | Performs Bitwise XOR on operands and assign the value to left operand | a ^= b |
| >>= | Performs Bitwise right shift on operands and assign the result to left operand | a >>= b |
| <<= | Performs Bitwise left shift on operands and assign the result to left operand | a <<= b |
| := | Assign a value to a variable within an expression | a := exp |
Here are the Assignment Operators in Python with examples.
Assignment Operator
Assignment Operators are used to assign values to variables. This operator is used to assign the value of the right side of the expression to the left side operand.
Addition Assignment Operator
The Addition Assignment Operator is used to add the right-hand side operand with the left-hand side operand and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the addition assignment operator which will first perform the addition operation and then assign the result to the variable on the left-hand side.
S ubtraction Assignment Operator
The Subtraction Assignment Operator is used to subtract the right-hand side operand from the left-hand side operand and then assigning the result to the left-hand side operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the subtraction assignment operator which will first perform the subtraction operation and then assign the result to the variable on the left-hand side.
M ultiplication Assignment Operator
The Multiplication Assignment Operator is used to multiply the right-hand side operand with the left-hand side operand and then assigning the result to the left-hand side operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the multiplication assignment operator which will first perform the multiplication operation and then assign the result to the variable on the left-hand side.

D ivision Assignment Operator
The Division Assignment Operator is used to divide the left-hand side operand with the right-hand side operand and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the division assignment operator which will first perform the division operation and then assign the result to the variable on the left-hand side.
M odulus Assignment Operator
The Modulus Assignment Operator is used to take the modulus, that is, it first divides the operands and then takes the remainder and assigns it to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the modulus assignment operator which will first perform the modulus operation and then assign the result to the variable on the left-hand side.
F loor Division Assignment Operator
The Floor Division Assignment Operator is used to divide the left operand with the right operand and then assigs the result(floor value) to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the floor division assignment operator which will first perform the floor division operation and then assign the result to the variable on the left-hand side.
Exponentiation Assignment Operator
The Exponentiation Assignment Operator is used to calculate the exponent(raise power) value using operands and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the exponentiation assignment operator which will first perform exponent operation and then assign the result to the variable on the left-hand side.
Bitwise AND Assignment Operator
The Bitwise AND Assignment Operator is used to perform Bitwise AND operation on both operands and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise AND assignment operator which will first perform Bitwise AND operation and then assign the result to the variable on the left-hand side.
Bitwise OR Assignment Operator
The Bitwise OR Assignment Operator is used to perform Bitwise OR operation on the operands and then assigning result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise OR assignment operator which will first perform bitwise OR operation and then assign the result to the variable on the left-hand side.
Bitwise XOR Assignment Operator
The Bitwise XOR Assignment Operator is used to perform Bitwise XOR operation on the operands and then assigning result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise XOR assignment operator which will first perform bitwise XOR operation and then assign the result to the variable on the left-hand side.
Bitwise Right Shift Assignment Operator
The Bitwise Right Shift Assignment Operator is used to perform Bitwise Right Shift Operation on the operands and then assign result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise right shift assignment operator which will first perform bitwise right shift operation and then assign the result to the variable on the left-hand side.
Bitwise Left Shift Assignment Operator
The Bitwise Left Shift Assignment Operator is used to perform Bitwise Left Shift Opertator on the operands and then assign result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise left shift assignment operator which will first perform bitwise left shift operation and then assign the result to the variable on the left-hand side.
Walrus Operator
The Walrus Operator in Python is a new assignment operator which is introduced in Python version 3.8 and higher. This operator is used to assign a value to a variable within an expression.
Example: In this code, we have a Python list of integers. We have used Python Walrus assignment operator within the Python while loop . The operator will solve the expression on the right-hand side and assign the value to the left-hand side operand ‘x’ and then execute the remaining code.
Assignment Operators in Python – FAQs
What are assignment operators in python.
Assignment operators in Python are used to assign values to variables. These operators can also perform additional operations during the assignment. The basic assignment operator is = , which simply assigns the value of the right-hand operand to the left-hand operand. Other common assignment operators include += , -= , *= , /= , %= , and more, which perform an operation on the variable and then assign the result back to the variable.
What is the := Operator in Python?
The := operator, introduced in Python 3.8, is known as the “walrus operator”. It is an assignment expression, which means that it assigns values to variables as part of a larger expression. Its main benefit is that it allows you to assign values to variables within expressions, including within conditions of loops and if statements, thereby reducing the need for additional lines of code. Here’s an example: # Example of using the walrus operator in a while loop while (n := int(input("Enter a number (0 to stop): "))) != 0: print(f"You entered: {n}") This loop continues to prompt the user for input and immediately uses that input in both the condition check and the loop body.
What is the Assignment Operator in Structure?
In programming languages that use structures (like C or C++), the assignment operator = is used to copy values from one structure variable to another. Each member of the structure is copied from the source structure to the destination structure. Python, however, does not have a built-in concept of ‘structures’ as in C or C++; instead, similar functionality is achieved through classes or dictionaries.
What is the Assignment Operator in Python Dictionary?
In Python dictionaries, the assignment operator = is used to assign a new key-value pair to the dictionary or update the value of an existing key. Here’s how you might use it: my_dict = {} # Create an empty dictionary my_dict['key1'] = 'value1' # Assign a new key-value pair my_dict['key1'] = 'updated value' # Update the value of an existing key print(my_dict) # Output: {'key1': 'updated value'}
What is += and -= in Python?
The += and -= operators in Python are compound assignment operators. += adds the right-hand operand to the left-hand operand and assigns the result to the left-hand operand. Conversely, -= subtracts the right-hand operand from the left-hand operand and assigns the result to the left-hand operand. Here are examples of both: # Example of using += a = 5 a += 3 # Equivalent to a = a + 3 print(a) # Output: 8 # Example of using -= b = 10 b -= 4 # Equivalent to b = b - 4 print(b) # Output: 6 These operators make code more concise and are commonly used in loops and iterative data processing.
Please Login to comment...
Similar reads.
- Python-Operators
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Get Started With Python
- Your First Python Program
- Python Comments
Python Fundamentals
- Python Variables and Literals
- Python Type Conversion
- Python Basic Input and Output
Python Operators
Python flow control.
Python if...else Statement
- Python for Loop
- Python while Loop
- Python break and continue
- Python pass Statement
Python Data types
- Python Numbers and Mathematics
- Python List
- Python Tuple
- Python String
- Python Dictionary
- Python Functions
- Python Function Arguments
- Python Variable Scope
- Python Global Keyword
- Python Recursion
- Python Modules
- Python Package
- Python Main function
Python Files
- Python Directory and Files Management
- Python CSV: Read and Write CSV files
- Reading CSV files in Python
- Writing CSV files in Python
- Python Exception Handling
- Python Exceptions
- Python Custom Exceptions
Python Object & Class
- Python Objects and Classes
- Python Inheritance
- Python Multiple Inheritance
- Polymorphism in Python
Python Operator Overloading
Python Advanced Topics
- List comprehension
- Python Lambda/Anonymous Function
- Python Iterators
- Python Generators
- Python Namespace and Scope
- Python Closures
- Python Decorators
- Python @property decorator
- Python RegEx
Python Date and Time
- Python datetime
- Python strftime()
- Python strptime()
- How to get current date and time in Python?
- Python Get Current Time
- Python timestamp to datetime and vice-versa
- Python time Module
- Python sleep()
Additional Topic
Precedence and Associativity of Operators in Python
- Python Keywords and Identifiers
- Python Asserts
- Python Json
- Python *args and **kwargs
Python Tutorials
Python 3 Tutorial
- Python Strings
- Python any()
Operators are special symbols that perform operations on variables and values. For example,
Here, + is an operator that adds two numbers: 5 and 6 .
- Types of Python Operators
Here's a list of different types of Python operators that we will learn in this tutorial.
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Special Operators
1. Python Arithmetic Operators
Arithmetic operators are used to perform mathematical operations like addition, subtraction, multiplication, etc. For example,
Here, - is an arithmetic operator that subtracts two values or variables.
Operator | Operation | Example |
---|---|---|
Addition | ||
Subtraction | ||
Multiplication | ||
Division | ||
Floor Division | ||
Modulo | ||
Power |
Example 1: Arithmetic Operators in Python
In the above example, we have used multiple arithmetic operators,
- + to add a and b
- - to subtract b from a
- * to multiply a and b
- / to divide a by b
- // to floor divide a by b
- % to get the remainder
- ** to get a to the power b
2. Python Assignment Operators
Assignment operators are used to assign values to variables. For example,
Here, = is an assignment operator that assigns 5 to x .
Here's a list of different assignment operators available in Python.
Operator | Name | Example |
---|---|---|
Assignment Operator | ||
Addition Assignment | ||
Subtraction Assignment | ||
Multiplication Assignment | ||
Division Assignment | ||
Remainder Assignment | ||
Exponent Assignment |
Example 2: Assignment Operators
Here, we have used the += operator to assign the sum of a and b to a .
Similarly, we can use any other assignment operators as per our needs.
3. Python Comparison Operators
Comparison operators compare two values/variables and return a boolean result: True or False . For example,
Here, the > comparison operator is used to compare whether a is greater than b or not.
Operator | Meaning | Example |
---|---|---|
Is Equal To | gives us | |
Not Equal To | gives us | |
Greater Than | gives us | |
Less Than | gives us | |
Greater Than or Equal To | give us | |
Less Than or Equal To | gives us |
Example 3: Comparison Operators
Note: Comparison operators are used in decision-making and loops . We'll discuss more of the comparison operator and decision-making in later tutorials.
4. Python Logical Operators
Logical operators are used to check whether an expression is True or False . They are used in decision-making. For example,
Here, and is the logical operator AND . Since both a > 2 and b >= 6 are True , the result is True .
Operator | Example | Meaning |
---|---|---|
a b | : only if both the operands are | |
a b | : if at least one of the operands is | |
a | : if the operand is and vice-versa. |
Example 4: Logical Operators
Note : Here is the truth table for these logical operators.
5. Python Bitwise operators
Bitwise operators act on operands as if they were strings of binary digits. They operate bit by bit, hence the name.
For example, 2 is 10 in binary, and 7 is 111 .
In the table below: Let x = 10 ( 0000 1010 in binary) and y = 4 ( 0000 0100 in binary)
Operator | Meaning | Example |
---|---|---|
Bitwise AND | x & y = 0 ( ) | |
Bitwise OR | x | y = 14 ( ) | |
Bitwise NOT | ~x = -11 ( ) | |
Bitwise XOR | x ^ y = 14 ( ) | |
Bitwise right shift | x >> 2 = 2 ( ) | |
Bitwise left shift | x 0010 1000) |
6. Python Special operators
Python language offers some special types of operators like the identity operator and the membership operator. They are described below with examples.
- Identity operators
In Python, is and is not are used to check if two values are located at the same memory location.
It's important to note that having two variables with equal values doesn't necessarily mean they are identical.
Operator | Meaning | Example |
---|---|---|
if the operands are identical (refer to the same object) | ||
if the operands are not identical (do not refer to the same object) |
Example 4: Identity operators in Python
Here, we see that x1 and y1 are integers of the same values, so they are equal as well as identical. The same is the case with x2 and y2 (strings).
But x3 and y3 are lists. They are equal but not identical. It is because the interpreter locates them separately in memory, although they are equal.
- Membership operators
In Python, in and not in are the membership operators. They are used to test whether a value or variable is found in a sequence ( string , list , tuple , set and dictionary ).
In a dictionary, we can only test for the presence of a key, not the value.
Operator | Meaning | Example |
---|---|---|
if value/variable is in the sequence | ||
if value/variable is in the sequence |
Example 5: Membership operators in Python
Here, 'H' is in message , but 'hello' is not present in message (remember, Python is case-sensitive).
Similarly, 1 is key, and 'a' is the value in dictionary dict1 . Hence, 'a' in y returns False .
- Precedence and Associativity of operators in Python
Table of Contents
- Introduction
- Python Arithmetic Operators
- Python Assignment Operators
- Python Comparison Operators
- Python Logical Operators
- Python Bitwise operators
- Python Special operators
Write a function to split the restaurant bill among friends.
- Take the subtotal of the bill and the number of friends as inputs.
- Calculate the total bill by adding 20% tax to the subtotal and then divide it by the number of friends.
- Return the amount each friend has to pay, rounded off to two decimal places.
Video: Operators in Python
Sorry about that.
Related Tutorials
Python Tutorial
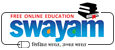
Programming, Data Structures And Algorithms Using Python
Course Status : | Completed | ||
Course Type : | Elective | ||
Duration : | 8 weeks | ||
Category : | |||
Credit Points : | 2 | Undergraduate | |
Start Date : | 14 Sep 2020 | ||
End Date : | 06 Nov 2020 | ||
Enrollment Ends : | 25 Sep 2020 | ||
Exam Date : | 18 Dec 2020 IST |
Operator | Example | Same As | Try it |
---|---|---|---|
= | x = 5 | x = 5 | |
+= | x += 3 | x = x + 3 | |
-= | x -= 3 | x = x - 3 | |
*= | x *= 3 | x = x * 3 | |
/= | x /= 3 | x = x / 3 | |
%= | x %= 3 | x = x % 3 | |
//= | x //= 3 | x = x // 3 | |
**= | x **= 3 | x = x ** 3 | |
&= | x &= 3 | x = x & 3 | |
|= | x |= 3 | x = x | 3 | |
^= | x ^= 3 | x = x ^ 3 | |
>>= | x >>= 3 | x = x >> 3 | |
<<= | x <<= 3 | x = x << 3 |
Related Pages

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

IMAGES
COMMENTS
These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges. All exercises are tested on Python 3. Each exercise has 10-20 Questions. The solution is provided for every question. These Python programming exercises are suitable for all Python developers.
In this way, Python can reverse a list of 3 numbers or 100 numbers or ten million numbers with the same instructions. Repetition is a key idea behind programming languages. This means that your task with these exercises is to determine a sequence of steps that solve the problem and then find the Python code that will run those instructions.
The best way to learn is by practising it more and more. The best thing about this Python practice exercise is that it helps you learn Python using sets of detailed programming questions from basic to advanced. It covers questions on core Python concepts as well as applications of Python in various domains.
The Exercise. We have gathered a variety of Python exercises from most of the chapters in our Python Tutorial. The exercises are a mix of "multiple choice" and "fill in the blanks" questions. The answer can be found in the corresponding tutorial chapter. If you're stuck, or answer wrong, you can try again or hit the "Show Answer" button to see ...
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
In this article, we are going to understand the concept of Multi-Line statements in the Python programming language. Statements in Python: In Python, a statement is a logical command that a Python interpreter can read and carry out. It might be an assignment statement or an expression in Python. Multi-line Statement in Python: In Python, the statem
There are 7 modules in this course. This course aims to teach everyone the basics of programming computers using Python. We cover the basics of how one constructs a program from a series of simple instructions in Python. The course has no pre-requisites and avoids all but the simplest mathematics. Anyone with moderate computer experience should ...
6.0001 Introduction to Computer Science and Programming in Python is intended for students with little or no programming experience. It aims to provide students with an understanding of the role computation can play in solving problems and to help students, regardless of their major, feel justifiably confident of their ability to write small programs that allow them to accomplish useful goals.
A Gentle Introduction to Programming Using Python. Menu. More Info Syllabus Calendar Readings Lectures Assignments Exams Related Resources Assignments ... assignment Programming Assignments. Download Course. Over 2,500 courses & materials Freely sharing knowledge with learners and educators around the world.
Module 1 • 11 hours to complete. This first module covers an intro to programming and the Python language. We'll start by downloading and installing the necessary tools to begin programming and writing code in Python. After learning how to print to the console, we'll get an understanding of Python's basic data types, and how to do ...
There are 4 modules in this course. This introductory course is designed for beginners and individuals with limited programming experience who want to embark on their software development or data science journey using Python. Throughout the course, learners will gain a solid understanding of algorithmic thinking, Python syntax, code testing ...
Ashwin Joy. I'm the face behind Pythonista Planet. I learned my first programming language back in 2015. Ever since then, I've been learning programming and immersing myself in technology.
Python essential exercise is to help Python beginners to quickly learn basic skills by solving the questions.When you complete each question, you get more familiar with a control structure, loops, string, and list in Python. ... Write a program to iterate the first 10 numbers, and in each iteration, print the sum of the current and previous number.
Python Program to Check If Two Strings are Anagram. Python Program to Capitalize the First Character of a String. Python Program to Compute all the Permutation of the String. Python Program to Create a Countdown Timer. Python Program to Count the Number of Occurrence of a Character in String.
Now that you know the basics of Python programming, be sure to check out the wide range of Python tutorials, video courses, and resources here at Real Python to continue building your skills. Conclusion. Learning how to use Python and get your programming skills to the next level is a worthwhile endeavor. Python is a popular, productive, and ...
Object-oriented programming (OOP) is a method of structuring a program by bundling related properties and behaviors into individual objects. In this tutorial, you'll learn the basics of object-oriented programming in Python. Conceptually, objects are like the components of a system. Think of a program as a factory assembly line of sorts.
As soon as you assign a value to a variable, the old value is lost. x: int = 42. print(x) x = 43. print(x) The assignment of a variable to another variable, for instance b = a does not imply that if a is reassigned then b changes as well. a: int = 42. b: int = a # a and b have now the same value. print('a =', a)
There are 5 modules in this course. In this course, you will be introduced to foundational programming skills with basic Python Syntax. You'll learn how to use code to solve problems. You'll dive deep into the Python ecosystem and learn popular modules, libraries and tools for Python. You'll also get hands-on with objects, classes and ...
Assignment Operator. Assignment Operators are used to assign values to variables. This operator is used to assign the value of the right side of the expression to the left side operand. Python. # Assigning values using # Assignment Operator a = 3 b = 5 c = a + b # Output print(c) Output. 8.
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
6. Python Special operators. Python language offers some special types of operators like the identity operator and the membership operator. They are described below with examples. Identity operators. In Python, is and is not are used to check if two values are located at the same memory location.
It goes on to cover searching and sorting algorithms, dynamic programming and backtracking, as well as topics such as exception handling and using files. As far as data structures are concerned, the course covers Python dictionaries as well as classes and objects for defining user defined datatypes such as linked lists and binary search trees.
Python Assignment Operators. Assignment operators are used to assign values to variables: Operator. Example. Same As. Try it. =. x = 5. x = 5.