Home » PHP Tutorial » PHP Assignment Operators

PHP Assignment Operators
Summary : in this tutorial, you will learn about the most commonly used PHP assignment operators.
Introduction to the PHP assignment operator
PHP uses the = to represent the assignment operator. The following shows the syntax of the assignment operator:
On the left side of the assignment operator ( = ) is a variable to which you want to assign a value. And on the right side of the assignment operator ( = ) is a value or an expression.
When evaluating the assignment operator ( = ), PHP evaluates the expression on the right side first and assigns the result to the variable on the left side. For example:
In this example, we assigned 10 to $x, 20 to $y, and the sum of $x and $y to $total.
The assignment expression returns a value assigned, which is the result of the expression in this case:
It means that you can use multiple assignment operators in a single statement like this:
In this case, PHP evaluates the right-most expression first:
The variable $y is 20 .
The assignment expression $y = 20 returns 20 so PHP assigns 20 to $x . After the assignments, both $x and $y equal 20.
Arithmetic assignment operators
Sometimes, you want to increase a variable by a specific value. For example:
How it works.
- First, $counter is set to 1 .
- Then, increase the $counter by 1 and assign the result to the $counter .
After the assignments, the value of $counter is 2 .
PHP provides the arithmetic assignment operator += that can do the same but with a shorter code. For example:
The expression $counter += 1 is equivalent to the expression $counter = $counter + 1 .
Besides the += operator, PHP provides other arithmetic assignment operators. The following table illustrates all the arithmetic assignment operators:
Operator | Example | Equivalent | Operation |
---|---|---|---|
+= | $x += $y | $x = $x + $y | Addition |
-= | $x -= $y | $x = $x – $y | Subtraction |
*= | $x *= $y | $x = $x * $y | Multiplication |
/= | $x /= $y | $x = $x / $y | Division |
%= | $x %= $y | $x = $x % $y | Modulus |
**= | $z **= $y | $x = $x ** $y | Exponentiation |
Concatenation assignment operator
PHP uses the concatenation operator (.) to concatenate two strings. For example:
By using the concatenation assignment operator you can concatenate two strings and assigns the result string to a variable. For example:
- Use PHP assignment operator ( = ) to assign a value to a variable. The assignment expression returns the value assigned.
- Use arithmetic assignment operators to carry arithmetic operations and assign at the same time.
- Use concatenation assignment operator ( .= )to concatenate strings and assign the result to a variable in a single statement.
- Language Reference
Assignment Operators
The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". Don't. It really means that the left operand gets set to the value of the expression on the right (that is, "gets set to").
The value of an assignment expression is the value assigned. That is, the value of " $a = 3 " is 3. This allows you to do some tricky things: <?php $a = ( $b = 4 ) + 5 ; // $a is equal to 9 now, and $b has been set to 4. ?>
In addition to the basic assignment operator, there are "combined operators" for all of the binary arithmetic , array union and string operators that allow you to use a value in an expression and then set its value to the result of that expression. For example: <?php $a = 3 ; $a += 5 ; // sets $a to 8, as if we had said: $a = $a + 5; $b = "Hello " ; $b .= "There!" ; // sets $b to "Hello There!", just like $b = $b . "There!"; ?>
Note that the assignment copies the original variable to the new one (assignment by value), so changes to one will not affect the other. This may also have relevance if you need to copy something like a large array inside a tight loop.
An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference. Objects may be explicitly copied via the clone keyword.
Assignment by Reference
Assignment by reference is also supported, using the " $var = &$othervar; " syntax. Assignment by reference means that both variables end up pointing at the same data, and nothing is copied anywhere.
Example #1 Assigning by reference
The new operator returns a reference automatically, as such assigning the result of new by reference is an error.
The above example will output:
More information on references and their potential uses can be found in the References Explained section of the manual.
Arithmetic Assignment Operators
Example | Equivalent | Operation |
---|---|---|
$a += $b | $a = $a + $b | Addition |
$a -= $b | $a = $a - $b | Subtraction |
$a *= $b | $a = $a * $b | Multiplication |
$a /= $b | $a = $a / $b | Division |
$a %= $b | $a = $a % $b | Modulus |
$a **= $b | $a = $a ** $b | Exponentiation |
Bitwise Assignment Operators
Example | Equivalent | Operation |
---|---|---|
$a &= $b | $a = $a & $b | Bitwise And |
$a |= $b | $a = $a | $b | Bitwise Or |
$a ^= $b | $a = $a ^ $b | Bitwise Xor |
$a <<= $b | $a = $a << $b | Left Shift |
$a >>= $b | $a = $a >> $b | Right Shift |
Other Assignment Operators
Example | Equivalent | Operation |
---|---|---|
$a .= $b | $a = $a . $b | String Concatenation |
$a ??= $b | $a = $a ?? $b | Null Coalesce |
- arithmetic operators
- bitwise operators
- null coalescing operator
Improve This Page
User contributed notes.

PHP Tutorial
Php advanced, mysql database, php examples, php reference, php operators.
Operators are used to perform operations on variables and values.
PHP divides the operators in the following groups:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Increment/Decrement operators
- Logical operators
- String operators
- Array operators
- Conditional assignment operators
PHP Arithmetic Operators
The PHP arithmetic operators are used with numeric values to perform common arithmetical operations, such as addition, subtraction, multiplication etc.
Operator | Name | Example | Result | Try it |
---|---|---|---|---|
+ | Addition | $x + $y | Sum of $x and $y | |
- | Subtraction | $x - $y | Difference of $x and $y | |
* | Multiplication | $x * $y | Product of $x and $y | |
/ | Division | $x / $y | Quotient of $x and $y | |
% | Modulus | $x % $y | Remainder of $x divided by $y | |
** | Exponentiation | $x ** $y | Result of raising $x to the $y'th power |
PHP Assignment Operators
The PHP assignment operators are used with numeric values to write a value to a variable.
The basic assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right.
Assignment | Same as... | Description | Try it |
---|---|---|---|
x = y | x = y | The left operand gets set to the value of the expression on the right | |
x += y | x = x + y | Addition | |
x -= y | x = x - y | Subtraction | |
x *= y | x = x * y | Multiplication | |
x /= y | x = x / y | Division | |
x %= y | x = x % y | Modulus |
Advertisement
PHP Comparison Operators
The PHP comparison operators are used to compare two values (number or string):
Operator | Name | Example | Result | Try it |
---|---|---|---|---|
== | Equal | $x == $y | Returns true if $x is equal to $y | |
=== | Identical | $x === $y | Returns true if $x is equal to $y, and they are of the same type | |
!= | Not equal | $x != $y | Returns true if $x is not equal to $y | |
<> | Not equal | $x <> $y | Returns true if $x is not equal to $y | |
!== | Not identical | $x !== $y | Returns true if $x is not equal to $y, or they are not of the same type | |
> | Greater than | $x > $y | Returns true if $x is greater than $y | |
< | Less than | $x < $y | Returns true if $x is less than $y | |
>= | Greater than or equal to | $x >= $y | Returns true if $x is greater than or equal to $y | |
<= | Less than or equal to | $x <= $y | Returns true if $x is less than or equal to $y | |
<=> | Spaceship | $x <=> $y | Returns an integer less than, equal to, or greater than zero, depending on if $x is less than, equal to, or greater than $y. Introduced in PHP 7. |
PHP Increment / Decrement Operators
The PHP increment operators are used to increment a variable's value.
The PHP decrement operators are used to decrement a variable's value.
Operator | Same as... | Description | Try it |
---|---|---|---|
++$x | Pre-increment | Increments $x by one, then returns $x | |
$x++ | Post-increment | Returns $x, then increments $x by one | |
--$x | Pre-decrement | Decrements $x by one, then returns $x | |
$x-- | Post-decrement | Returns $x, then decrements $x by one |
PHP Logical Operators
The PHP logical operators are used to combine conditional statements.
Operator | Name | Example | Result | Try it |
---|---|---|---|---|
and | And | $x and $y | True if both $x and $y are true | |
or | Or | $x or $y | True if either $x or $y is true | |
xor | Xor | $x xor $y | True if either $x or $y is true, but not both | |
&& | And | $x && $y | True if both $x and $y are true | |
|| | Or | $x || $y | True if either $x or $y is true | |
! | Not | !$x | True if $x is not true |
PHP String Operators
PHP has two operators that are specially designed for strings.
Operator | Name | Example | Result | Try it |
---|---|---|---|---|
. | Concatenation | $txt1 . $txt2 | Concatenation of $txt1 and $txt2 | |
.= | Concatenation assignment | $txt1 .= $txt2 | Appends $txt2 to $txt1 |
PHP Array Operators
The PHP array operators are used to compare arrays.
Operator | Name | Example | Result | Try it |
---|---|---|---|---|
+ | Union | $x + $y | Union of $x and $y | |
== | Equality | $x == $y | Returns true if $x and $y have the same key/value pairs | |
=== | Identity | $x === $y | Returns true if $x and $y have the same key/value pairs in the same order and of the same types | |
!= | Inequality | $x != $y | Returns true if $x is not equal to $y | |
<> | Inequality | $x <> $y | Returns true if $x is not equal to $y | |
!== | Non-identity | $x !== $y | Returns true if $x is not identical to $y |
PHP Conditional Assignment Operators
The PHP conditional assignment operators are used to set a value depending on conditions:
Operator | Name | Example | Result | Try it |
---|---|---|---|---|
?: | Ternary | $x = ? : | Returns the value of $x. The value of $x is if = TRUE. The value of $x is if = FALSE | |
?? | Null coalescing | $x = ?? | Returns the value of $x. The value of $x is if exists, and is not NULL. If does not exist, or is NULL, the value of $x is . Introduced in PHP 7 |

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- TutorialKart
- SAP Tutorials
- Salesforce Admin
- Salesforce Developer
- Visualforce
- Informatica
- Kafka Tutorial
- Spark Tutorial
- Tomcat Tutorial
- Python Tkinter
Programming
- Bash Script
- Julia Tutorial
- CouchDB Tutorial
- MongoDB Tutorial
- PostgreSQL Tutorial
- Android Compose
- Flutter Tutorial
- Kotlin Android
Web & Server
- Selenium Java
- PHP Tutorial
- PHP Install
- PHP Hello World
- Check if variable is set
- Convert string to int
- Convert string to float
- Convert string to boolean
- Convert int to string
- Convert int to float
- Get type of variable
- Subtraction
- Multiplication
- Exponentiation
- Simple Assignment
- Addition Assignment
- Subtraction Assignment
- ADVERTISEMENT
- Multiplication Assignment
- Division Assignment
- Modulus Assignment
- PHP Comments
- Python If AND
- Python If OR
- Python If NOT
- Do-While Loop
- Nested foreach
- echo() vs print()
- printf() vs sprintf()
- PHP Strings
- Create a string
- Create empty string
- Compare strings
- Count number of words in string
- Get ASCII value of a character
- Iterate over characters of a string
- Iterate over words of a string
- Print string to console
- String length
- Substring of a string
- Check if string is empty
- Check if strings are equal
- Check if strings are equal ignoring case
- Check if string contains specified substring
- Check if string starts with specific substring
- Check if string ends with specific substring
- Check if string starts with specific character
- Check if string starts with http
- Check if string starts with a number
- Check if string starts with an uppercase
- Check if string starts with a lowercase
- Check if string ends with a punctuation
- Check if string ends with forward slash (/) character
- Check if string contains number(s)
- Check if string contains only alphabets
- Check if string contains only numeric digits
- Check if string contains only lowercase
- Check if string contains only uppercase
- Check if string value is a valid number
- Check if string is a float value
- Append a string with suffix string
- Prepend a string with prefix string
- Concatenate strings
- Concatenate string and number
- Insert character at specific index in string
- Insert substring at specific index in string
- Repeat string for N times
- Replace substring
- Replace first occurrence
- Replace last occurrence
- Trim spaces from edges of string
- Trim specific characters from edges of the string
- Split string
- Split string into words
- Split string by comma
- Split string by single space
- Split string by new line
- Split string by any whitespace character
- Split string by one or more whitespace characters
- Split string into substrings of specified length
- Transformations
- Reverse a string
- Convert string to lowercase
- Convert string to uppercase
- Join elements of string array with separator string
- Delete first character of string
- Delete last character of string
- Count number of occurrences of substring in a string
- Count alphabet characters in a string
- Find index of substring in a string
- Find index of last occurrence in a string
- Find all the substrings that match a pattern
- Check if entire string matches a regular expression
- Check if string has a match for regular expression
- Sort array of strings
- Sort strings in array based on length
- Foramatting
- PHP – Format string
- PHP – Variable inside a string
- PHP – Parse JSON String
- Conversions
- PHP – Convert CSV String into Array
- PHP – Convert string array to CSV string
- Introduction to Arrays
- Indexed arrays
- Associative arrays
- Multi-dimensional arrays
- String array
- Array length
- Create Arrays
- Create indexed array
- Create associative array
- Create empty array
- Check if array is empty
- Check if specific element is present in array .
- Check if two arrays are equal
- Check if any two adjacent values are same
- Read/Access Operations
- Access array elements using index
- Iterate through array using For loop
- Iterate over key-value pairs using foreach
- Array foreach()
- Get first element in array
- Get last element in array
- Get keys of array
- Get index of a key in array
- Find Operations
- Count occurrences of specific value in array
- Find index of value in array
- Find index of last occurrence of value in array
- Combine two arrays to create an Associative Array
- Convert array to string
- Convert array to CSV string
- Join array elements
- Reverse an array
- Split array into chunks
- Slice array by index
- Slice array by key
- Preserve keys while slicing array
- Truncate array
- Remove duplicate values in array
- Filter elements in array based on a condition
- Filter positive numbers in array
- Filter negative numbers in array
- Remove empty strings in array
- Delete Operations
- Delete specific value from array
- Delete value at specific index in array
- Remove first value in array
- Remove last value in array
- Array - Notice: Undefined offset: 0
- JSON encode
- Array Functions
- array_chunk()
- array_column()
- array_combine()
- array_change_key_case()
- array_count_values()
- array_diff_assoc()
- array_diff_key()
- array_diff_uassoc()
- array_diff_ukey()
- array_diff()
- array_fill()
- array_fill_keys()
- array_flip()
- array_key_exists()
- array_keys()
- array_pop()
- array_product()
- array_push()
- array_rand()
- array_replace()
- array_sum()
- array_values()
- Create an object or instance
- Constructor
- Constants in class
- Read only properties in class
- Encapsulation
- Inheritance
- Define property and method with same name in class
- Uncaught Error: Cannot access protected property
- Nested try catch
- Multiple catch blocks
- Catch multiple exceptions in a single catch block
- Print exception message
- Throw exception
- User defined Exception
- Get current timestamp
- Get difference between two given dates
- Find number of days between two given dates
- Add specific number of days to given date
- ❯ PHP Tutorial
- ❯ PHP Operators
- ❯ Assignment
PHP Assignment Operators
In this tutorial, you shall learn about Assignment Operators in PHP, different Assignment Operators available in PHP, their symbols, and how to use them in PHP programs, with examples.
Assignment Operators
Assignment Operators are used to perform to assign a value or modified value to a variable.
Assignment Operators Table
The following table lists out all the assignment operators in PHP programming.
Operator | Symbol | Example | Description |
---|---|---|---|
Simple Assignment | = | x = 5 | Assigns value of 5 to variable x. |
Addition Assignment | += | Assigns the result of to x. | |
Subtraction Assignment | -= | Assigns the result of to x. | |
Multiplication Assignment | *= | Assigns the result of to x. | |
Division Assignment | /= | Assigns the result of to x. | |
Modulus Assignment | %= | Assigns the result of to x. |
In the following program, we will take values in variables $x and $y , and perform assignment operations on these values using PHP Assignment Operators.
PHP Program
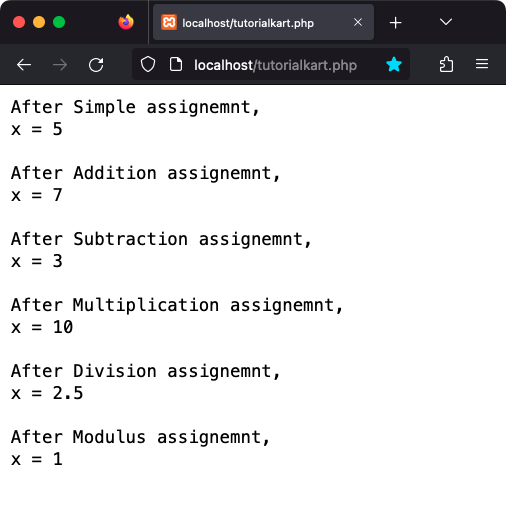
Assignment Operators Tutorials
The following tutorials cover each of the Assignment Operators in PHP in detail with examples.
- PHP Simple Assignment
- PHP Addition Assignment
- PHP Subtraction Assignment
- PHP Multiplication Assignment
- PHP Division Assignment
- PHP Modulus Assignment
In this PHP Tutorial , we learned about all the Assignment Operators in PHP programming, with examples.

Popular Courses by TutorialKart
App developement, web development, online tools.
Assignment Operators
The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". Don't. It really means that the left operand gets set to the value of the expression on the right (that is, "gets set to").
The value of an assignment expression is the value assigned. That is, the value of " $a = 3 " is 3. This allows you to do some tricky things: <?php $a = ( $b = 4 ) + 5 ; // $a is equal to 9 now, and $b has been set to 4. ?>
For arrays , assigning a value to a named key is performed using the "=>" operator. The precedence of this operator is the same as other assignment operators.
In addition to the basic assignment operator, there are "combined operators" for all of the binary arithmetic , array union and string operators that allow you to use a value in an expression and then set its value to the result of that expression. For example: <?php $a = 3 ; $a += 5 ; // sets $a to 8, as if we had said: $a = $a + 5; $b = "Hello " ; $b .= "There!" ; // sets $b to "Hello There!", just like $b = $b . "There!"; ?>
Note that the assignment copies the original variable to the new one (assignment by value), so changes to one will not affect the other. This may also have relevance if you need to copy something like a large array inside a tight loop.
An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference in PHP 5. Objects may be explicitly copied via the clone keyword.
Assignment by Reference
Assignment by reference is also supported, using the " $var = &$othervar; " syntax. Assignment by reference means that both variables end up pointing at the same data, and nothing is copied anywhere.
Example #1 Assigning by reference
As of PHP 5, the new operator returns a reference automatically, so assigning the result of new by reference results in an E_DEPRECATED message in PHP 5.3 and later, and an E_STRICT message in earlier versions.
For example, this code will result in a warning: <?php class C {} /* The following line generates the following error message: * Deprecated: Assigning the return value of new by reference is deprecated in... */ $o = &new C ; ?>
More information on references and their potential uses can be found in the References Explained section of the manual.
PHP.net news & announcements
- 2024-08-29 PHP 8.3.11 Released!
- 2024-08-29 PHP 8.4.0 Beta 4 now available for testing
- 2024-08-29 PHP 8.2.23 Released!
- 2024-08-27 API Platform Conference 2024
- 2024-08-15 PHP Russia 2024
PHP.net releases
- 2024-08-29 PHP 8.3.11 released!
- 2024-08-29 PHP 8.2.23 released!
- 2024-06-06 PHP 8.1.29 released!
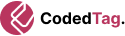
- Assignment Operators
PHP assignment operators enable you to frequently engage in performing calculations and operations on variables, requiring the assignment of results to other variables. Consequently, this is precisely where assignment operators prove indispensable, allowing you to seamlessly execute an operation and assign the result to a variable within a single statement.
In the following sections, we’ll delve into the different types of PHP assignment operators and explore how to use them.
Table of Contents
Php arithmetic assignment operators, php bitwise assignment operators.
- Null Coalescing Operator
Assigning a Reference to a PHP Variable
Other assignment operators, wrapping up.
The most commonly used assignment operator in PHP is the equals sign (=). For instance, in the following code, the value 10 is assigned to the variable $x:
Now, let’s explore each type with examples:
Numeric data undergoes mathematical operations through the utilization of arithmetic operators. In PHP, this is where arithmetic assignment operators come into play, employed to perform these operations. The arithmetic assignment operators include:
- += or $x + $y (Addition Assignment Operator)
- -= or $x - $y (Subtraction Assignment Operator)
- *= or $x * $y (Multiplication Assignment Operator)
- /= or $x / $y (Division Assignment Operator)
- %= or $x % $y (Modulus Assignment Operator)
- **= or $x ** $y (Exponentiation Assignment Operator)
Consider the following example:
In this example, the addition assignment operator increases the value of $x by 5, and then assigns the result back to $x, producing an output of 15.
You can leverage these arithmetic assignment operators to perform complex calculations in a single statement, making your code more concise and easier to read. For more details, refer to this tutorial .
One crucial aspect of computer science involves the manipulation of binary bits in PHP. Let’s delve into one of the more complex assignment operators—specifically, the PHP bitwise operators.
Developers use bitwise operators to manipulate data at the bit level. PHP bitwise assignment operators perform bitwise operations. Here are the bitwise assignment operators:
- &= or $x & $y (Bitwise AND Assignment Operator)
- |= or $x | $y (Bitwise OR Assignment Operator)
- ^= or $x ^ $y (Bitwise XOR Assignment Operator)
- ~= or $x ~ $y (Bitwise NOT Assignment Operator)
- <<= or $x << $y (Left Shift Assignment Operator)
- >>= or $x >> $y (Right Shift Assignment Operator)
Let’s illustrate with an example:
In this example, a bitwise OR operation is performed between the values of $x and 2 using the bitwise OR assignment operator. The result is then assigned to $x, yielding an output of 7.
Here is a full explanation along with more examples of bitwise operators .
Let’s move to the section below to understand the Null Coalescing Operator in PHP.
Furthermore, developers use the null coalescing operator to assign a default value to a variable if it is null. The double question mark (??) symbolizes the null coalescing operator. Consider the following example:
In this example, the null coalescing operator is used to assign the value ‘John Doe’ to the variable $fullName. If $name is null, the value ‘John Doe’ will be assigned to $fullName.
The null coalescing operator simplifies code by enabling the assignment of default values to variables. By reading this tutorial , you will gain more information about it
Anyway, assigning a reference in PHP is one of the language’s benefits, enabling developers to set a value and refer back to it anywhere during the script-writing process. Let’s move to the section below to take a closer look at this concept.
Furthermore, individuals use the reference assignment operator =& to assign a reference to a variable rather than copying the value of the variable. Consider the following example:
In this example, a reference to the variable $x is created using the reference assignment operator. The value 10 is then assigned to $x, and the output displays the value of $y. Since $y is a reference to $x, it also changes to 10, resulting in an output of 10.
The reference assignment operator proves useful when working with large data sets, enabling you to avoid copying large amounts of data.
Let’s explore some other assignment operators in the paragraphs below.
In addition to the arithmetic, bitwise, null coalescing, and reference assignment operators, PHP provides other assignment operators for specific use cases. These operators are:
- .= (Concatenation Assignment Operator)
- ??= (Null Coalescing Assignment Operator)
Now, let’s explore each of these operators in turn:
Concatenation Assignment Operator: Used to concatenate a string onto the end of another string. For example:
In this example, the concatenation assignment operator appends the value of $string2 to the end of $string1, resulting in the output “Hello World!”.
The Null Coalescing Assignment Operator is employed to assign a default value to a variable when it is detected as null. For example:
In this example, the null coalescing assignment operator assigns the value ‘John Doe’ to the variable $name. If $name is null, the value ‘John Doe’ will be assigned to $name.
Let’s summarize it.
we’ve taken a comprehensive look at the different types of PHP assignment operators and how to use them. Assignment operators are essential tools for any PHP developer, facilitating calculations and operations on variables while assigning results to other variables in a single statement.
Moreover, leveraging assignment operators allows you to make your code more concise, easier to read, and helps in avoiding common programming errors.
Did you find this article helpful?
Sorry about that. How can we improve it ?
- Facebook -->
- Twitter -->
- Linked In -->
- Install PHP
- Hello World
- PHP Constant
- PHP Comments
PHP Functions
- Parameters and Arguments
- Anonymous Functions
- Variable Function
- Arrow Functions
- Variadic Functions
- Named Arguments
- Callable Vs Callback
- Variable Scope
Control Structures
- If-else Block
- Break Statement
PHP Operators
- Operator Precedence
- PHP Arithmetic Operators
- PHP Bitwise Operators
- PHP Comparison Operators
- PHP Increment and Decrement Operator
- PHP Logical Operators
- PHP String Operators
- Array Operators
- Conditional Operators
- Ternary Operator
- PHP Enumerable
- PHP NOT Operator
- PHP OR Operator
- PHP Spaceship Operator
- AND Operator
- Exclusive OR
- Spread Operator
Data Format and Types
- PHP Data Types
- PHP Type Juggling
- PHP Type Casting
- PHP strict_types
- Type Hinting
- PHP Boolean Type
- PHP Iterable
- PHP Resource
- Associative Arrays
- Multidimensional Array
String and Patterns
- Remove the Last Char
Other Tutorials
- If Condition
- require_once & require
- array_map()
- Elvis Operator
- Predefined Constants
- PHP History
PHP Assignment Operators
Php tutorial index.
PHP assignment operators applied to assign the result of an expression to a variable. = is a fundamental assignment operator in PHP. It means that the left operand gets set to the value of the assignment expression on the right.
Operator | Description |
---|---|
= | Assign |
+= | Increments then assigns |
-= | Decrements then assigns |
*= | Multiplies then assigns |
/= | Divides then assigns |
%= | Modulus then assigns |
- ▼PHP Operators
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Assignment Operators
- Bitwise Operators
- String Operators
- Array Operators
- Incrementing Decrementing Operators
PHP Assignment Operators
Description.
Assignment operators allow writing a value to a variable . The first operand must be a variable and the basic assignment operator is "=". The value of an assignment expression is the final value assigned to the variable. In addition to the regular assignment operator "=", several other assignment operators are composites of an operator followed by an equal sign.
Interestingly all five arithmetic operators have corresponding assignment operators, Here is the list.
The following table discussed more details of the said assignment operators.
Shorthand | Expression | Description |
---|---|---|
$a+= $b | $a = $a + $b | Adds 2 numbers and assigns the result to the first. |
$a-= $b | $a = $a -$b | Subtracts 2 numbers and assigns the result to the first. |
$a*= $b | $a = $a*$b | Multiplies 2 numbers and assigns the result to the first. |
$a/= $b | $a = $a/$b | Divides 2 numbers and assigns the result to the first. |
$a%= $b | $a = $a%$b | Computes the modulus of 2 numbers and assigns the result to the first. |
View the example in the browser
Previous: Logical Operators Next: Bitwise Operators
Follow us on Facebook and Twitter for latest update.
- Weekly Trends and Language Statistics
- PHP - Introduction
- PHP - Syntax
- PHP - Comments
- PHP - Variables
- PHP - Constants
- PHP - Data Types
- PHP - Operators
- PHP - Echo and Print Statements
- PHP - Strings
- PHP - If Else
- PHP - Switch
- PHP - While Loop
- PHP - For Loop
- PHP - Foreach Loop
- PHP - goto Statement
- PHP - Continue Statement
- PHP - Break Statement
- PHP - Arrays
- PHP - Associative Arrays
- PHP - Sorting Arrays
- PHP - Functions
- PHP - Scope of Variables
- PHP - GET & POST
- PHP - Predefined Variables
- PHP - Date & time
- PHP - Cookies
- PHP - Classes/Objects
- PHP - Constructors
- PHP - Destructors
- PHP - Encapsulation
- PHP - Inheritance
- PHP - Exceptions
- PHP - Error Handling
- PHP - Sending Emails
- PHP & MySQL
- PHP - Built-In Functions
- PHP - Data Structures
- PHP - Examples
- PHP - Interview Questions
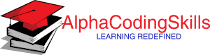

- Programming Languages
- Web Technologies
- Database Technologies
- Microsoft Technologies
- Python Libraries
- Data Structures
- Interview Questions
- C++ Standard Library
- C Standard Library
- Java Utility Library
- Java Default Package
- PHP Function Reference
PHP - assignment operators example
The example below shows the usage of assignment and compound assignment operators:
- = Assignment operator
- += Addition AND assignment operator
- -= Subtraction AND assignment operator
- *= Multiply AND assignment operator
- /= Division AND assignment operator
- **= Exponent AND assignment operator
- %= Modulo AND assignment operator
The output of the above code will be:
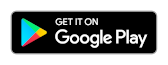
- Data Structures Tutorial
- Algorithms Tutorial
- JavaScript Tutorial
- Python Tutorial
- MySQLi Tutorial
- Java Tutorial
- Scala Tutorial
- C++ Tutorial
- C# Tutorial
- PHP Tutorial
- MySQL Tutorial
- SQL Tutorial
- PHP Function reference
- C++ - Standard Library
- Java.lang Package
- Ruby Tutorial
- Rust Tutorial
- Swift Tutorial
- Perl Tutorial
- HTML Tutorial
- CSS Tutorial
- AJAX Tutorial
- XML Tutorial
- Online Compilers
- QuickTables
- NumPy Tutorial
- Pandas Tutorial
- Matplotlib Tutorial
- SciPy Tutorial
- Seaborn Tutorial
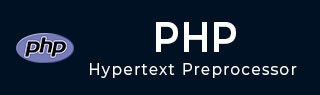
- PHP Tutorial
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook and Paypal Integration
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
- PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - Callbacks
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
PHP - Assignment Operators Examples
You can use assignment operators in PHP to assign values to variables. Assignment operators are shorthand notations to perform arithmetic or other operations while assigning a value to a variable. For instance, the "=" operator assigns the value on the right-hand side to the variable on the left-hand side.
Additionally, there are compound assignment operators like +=, -= , *=, /=, and %= which combine arithmetic operations with assignment. For example, "$x += 5" is a shorthand for "$x = $x + 5", incrementing the value of $x by 5. Assignment operators offer a concise way to update variables based on their current values.
The following table highligts the assignment operators that are supported by PHP −
Operator | Description | Example |
---|---|---|
= | Simple assignment operator. Assigns values from right side operands to left side operand | C = A + B will assign value of A + B into C |
+= | Add AND assignment operator. It adds right operand to the left operand and assign the result to left operand | C += A is equivalent to C = C + A |
-= | Subtract AND assignment operator. It subtracts right operand from the left operand and assign the result to left operand | C -= A is equivalent to C = C - A |
*= | Multiply AND assignment operator. It multiplies right operand with the left operand and assign the result to left operand | C *= A is equivalent to C = C * A |
/= | Divide AND assignment operator. It divides left operand with the right operand and assign the result to left operand | C /= A is equivalent to C = C / A |
%= | Modulus AND assignment operator. It takes modulus using two operands and assign the result to left operand | C %= A is equivalent to C = C % A |
The following example shows how you can use these assignment operators in PHP −
It will produce the following output −
- Language Reference
- Classes and Objects
Objects and references
One of the key-points of PHP OOP that is often mentioned is that "objects are passed by references by default". This is not completely true. This section rectifies that general thought using some examples.
A PHP reference is an alias, which allows two different variables to write to the same value. In PHP, an object variable doesn't contain the object itself as value. It only contains an object identifier which allows object accessors to find the actual object. When an object is sent by argument, returned or assigned to another variable, the different variables are not aliases: they hold a copy of the identifier, which points to the same object.
Example #1 References and Objects
The above example will output:
Improve This Page
User contributed notes 17 notes.

- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Are multiple variable assignments done by value or reference?
In the above code, are both $a and $b assigned the value of 0 , or is $a just referencing $b ?
- variable-assignment
8 Answers 8
With raw types this is a copy.
With objects though, that is another story (PHP 5)
- 9 Note, that the example is misleading: Changing the property of an object is not the same as changing the variable the object is referenced from. Because the variable never changed, it is of course the same. – KingCrunch Commented Dec 30, 2011 at 19:00
- 1 According to documentation , PHP uses a copy on write system. It means that with primitive types such as strings or ints are really created in memory on change. That's why, contrary to what we might think, creating references to optimize performance on small variables may not bring the desired effect, because PHP needs to create it before. However, objects are passed by reference by default since PHP5. – bgondy Commented Apr 11, 2013 at 17:00
- 4 The assign by reference with objects can be changed to assign by copy using clone . EG: $a = clone $b = new Obj; – Will B. Commented Mar 31, 2016 at 21:17
- At the class variable declaration level how it would be ? Like this private $_name = $address = ""; – Chaminda Bandara Commented Nov 14, 2016 at 10:12
Regard this code as:
The expression $b = 0 not only assigns 0 to $b , but it yields a result as well. That result is the right part of the assignment, or simply the value that $b got assigned to.
So, $a gets assigned 0 as well.
You could have tried it yourself
(currently I dont really care :D)
Thus: No, they are both independent variables with the value 0 .
- In that example, wouldn't you have to assign 5 to $b on the second line to prove the reference was not present? and yes, you are correct. I figured having the question up here would be nice for others searching as well though... – Evil Elf Commented Jun 6, 2011 at 19:47
- @Evil: Don't want to think about it, so added the other way round too. Both return 0 , thus it must be true in any case :) – KingCrunch Commented Jun 6, 2011 at 19:49
I'll recommend a good read on this: http://terriswallow.com/weblog/2007/multiple-and-dynamic-variable-assignment-in-php/ . In one of comments, you can read:
It should be noted that if you use multiple assignment on one line to assign an object, the object is assigned by reference. Therefore, if you change the value of the object’s property using either variable, the value essentially changes in both.
So I'll personally recommend that you assign the variables separately.
For the record:
So the conclusion is that there is no reference for primitives, but there IS a reference to objects.

- we proved this false actually with some tests. – Evil Elf Commented Jun 6, 2011 at 20:15
It depends what're you assigning.
If you're assigning a value, then the assignment copies the original variable to the new one.
Above code will return 0 as it's assignment by value.
An exception to the usual assignment by value behaviour within PHP occurs with objects, which are assigned by reference in PHP 5 automatically. Objects may be explicitly copied via the clone keyword.
Above code will print <html></html> .
Both $a and $b are assigned that value of 0. If you wanted $a to reference $b, you would preempt it with an ampersand, e.g.:
http://php.net/manual/en/language.oop5.basic.php
its assigns them both the value of 0
is the same as
Setting a variable equal to an existing object in PHP will pass by REFERENCE. It will not do a deep copy unless you add the clone keyword.
Long chains
This pattern applies to longer chains too.
You can confirm using a PHP sandbox: https://onlinephp.io/c/7b70f

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged php variables variable-assignment or ask your own question .
- The Overflow Blog
- The hidden cost of speed
- The creator of Jenkins discusses CI/CD and balancing business with open source
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
Hot Network Questions
- Replacing jockey wheels on Shimano Deore rear derailleur
- Where is this railroad track as seen in Rocky II during the training montage?
- Did the Turkish defense industry ever receive any significant foreign investment from any other OECD country?
- Hashable and ordered enums to describe states of a process
- Issue with the roots of the Equation of Time
- Riemannian metric for which arbitary path becomes a geodesic
- An error in formula proposed by Riley et al to calculate the sample size
- Why is LiCl a hypovalent covalent molecule?
- Does the average income in the US drop by $9,500 if you exclude the ten richest Americans?
- Deleting all files but some on Mac in Terminal
- Why would autopilot be prohibited below 1000 AGL?
- Why isn't a confidence level of anything >50% "good enough"?
- How to change upward facing track lights 26 feet above living room?
- Should on use mutate or insert to update storage?
- In which town of Europe (Germany ?) were this 2 photos taken during WWII?
- Why is it spelled "dummy" and not "dumby?"
- Why didn't Air Force Ones have camouflage?
- Sylvester primes
- What was the first "Star Trek" style teleporter in SF?
- How do you tip cash when you don't have proper denomination or no cash at all?
- How rich is the richest person in a society satisfying the Pareto principle?
- Why does the church of latter day saints not recognize the obvious sin of the angel Moroni according to the account of Joseph Smith's own words?
- how did the Apollo 11 know its precise gyroscopic position?
- DateTime.ParseExact returns today if date string and format are set to "General"

COMMENTS
Function Reference Affecting PHP's Behaviour Audio Formats Manipulation Authentication Services Command Line Specific Extensions Compression and Archive Extensions ... In addition to the basic assignment operator, there are "combined operators" for all of the binary arithmetic, array union and string operators that allow you to use a value in ...
It's called assignment by reference, which, to quote the manual, "means that both variables end up pointing at the same data, and nothing is copied anywhere". The only thing that is deprecated with =& is "assigning the result of new by reference" in PHP 5 , which might be the source of any confusion.
Use PHP assignment operator (=) to assign a value to a variable. The assignment expression returns the value assigned. Use arithmetic assignment operators to carry arithmetic operations and assign at the same time. Use concatenation assignment operator (.=)to concatenate strings and assign the result to a variable in a single statement.
PHP Manual; Language Reference; Operators; Change language: Assignment Operators. The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". Don't. It really means that the left operand gets set to the value of the expression on the right (that is, "gets set to"). ... In addition to the basic ...
The PHP assignment operators are used with numeric values to write a value to a variable. The basic assignment operator in PHP is "=". It means that the left operand gets set to the value of the assignment expression on the right. ... PHP Reference HTML Colors Java Reference Angular Reference jQuery Reference. Top Examples HTML Examples CSS ...
An operator is something that takes one or more values (or expressions, in programming jargon) and yields another value (so that the construction itself becomes an expression). Operators can be grouped according to the number of values they take. Unary operators take only one value, for example ! (the logical not operator) or ++ (the increment ...
Assignment operators are foundational to PHP programming, allowing developers to initialize, update, and manipulate variables efficiently. From the basic = operator to combined assignment operators like += and .=, understanding their proper use is crucial for writing clear and effective code. Encouragement to practice using different types of ...
In this tutorial, you shall learn about Assignment Operators in PHP, different Assignment Operators available in PHP, their symbols, and how to use them in PHP programs, with examples. Assignment Operators. Assignment Operators are used to perform to assign a value or modified value to a variable. Assignment Operators Table
The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". Don't. ... An exception to the usual assignment by value behaviour within PHP occurs with object s, which are assigned by reference in PHP 5. Objects may be explicitly copied via the clone keyword. Assignment by Reference.
The null coalescing operator simplifies code by enabling the assignment of default values to variables. By reading this tutorial, you will gain more information about it. Anyway, assigning a reference in PHP is one of the language's benefits, enabling developers to set a value and refer back to it anywhere during the script-writing process.
PHP Assignment Operators. PHP assignment operators applied to assign the result of an expression to a variable. = is a fundamental assignment operator in PHP. It means that the left operand gets set to the value of the assignment expression on the right. Operator.
Description. Assignment operators allow writing a value to a variable. The first operand must be a variable and the basic assignment operator is "=". The value of an assignment expression is the final value assigned to the variable. In addition to the regular assignment operator "=", several other assignment operators are composites of an ...
This is not the case. In fact, a C pointer version of this example (shown below) would behave exactly the same way (it would not modify what bar references) as the PHP reference version. int baz = 5; int* bar; void foo(int* var) {var = &baz;} foo(bar); In this case, just as in the case of PHP references, the call foo(bar) doesn't change what ...
The example below shows the usage of assignment and compound assignment operators: Assignment operator, Addition AND assignment operator, Subtraction AND ... PHP Tutorial. PHP - Home; PHP - Introduction; PHP - Syntax; PHP - Comments; ... PHP References. PHP - Built-In Functions; PHP - Data Structures; PHP - Examples; PHP - Interview Questions;
Example. =. Simple assignment operator. Assigns values from right side operands to left side operand. C = A + B will assign value of A + B into C. +=. Add AND assignment operator. It adds right operand to the left operand and assign the result to left operand. C += A is equivalent to C = C + A.
PHP Manual; Language Reference; Operators; Assignment Operators. The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". Don't. ... In addition to the basic assignment operator, there are "combined operators" for all of the binary arithmetic, array union and string operators that allow you to use a ...
Operators Control Structures Functions Classes and Objects ... however, that references inside arrays are potentially dangerous. Doing a normal (not by reference) assignment with a reference on the right side does not turn the left side into a reference, but references inside arrays are preserved in these normal assignments. ... is that PHP use ...
Assignment Operators The basic assignment operator is "=". Your first inclination might be to think of this as "equal to". Don't. It really means that the left ...
In other words, the ternary operator syntax is: condition ? do_if_condition_evaluates_to_true : do_if_evaluates_to_false; - Meekelis Commented Aug 26, 2018 at 9:58
Objects and references. ¶. One of the key-points of PHP OOP that is often mentioned is that "objects are passed by references by default". This is not completely true. This section rectifies that general thought using some examples. A PHP reference is an alias, which allows two different variables to write to the same value.
It means that with primitive types such as strings or ints are really created in memory on change. That's why, contrary to what we might think, creating references to optimize performance on small variables may not bring the desired effect, because PHP needs to create it before. However, objects are passed by reference by default since PHP5.